栈-顺序表中的public应该怎么编写
时间: 2024-09-29 20:10:29 浏览: 26
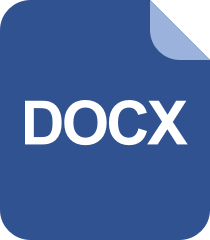
顺序栈与链栈的实现
栈是一种特殊的数据结构,它遵循“后进先出”(Last In First Out, LIFO)的原则,通常用于函数调用、表达式求值等场景。在顺序表中实现栈,你可以创建一个类,包含基本的操作方法如压栈(push)、弹栈(pop)、查看顶部元素(peek)以及判断是否为空(isEmpty)。在`public`访问修饰符下,这些方法应该设计成易于理解和使用的:
```java
// 假设Stack是一个名为Stack的类,E是元素类型
public class Stack<E> {
private int top; // 栈顶指针
private E[] elements; // 底层的顺序表
// 构造函数初始化大小
public Stack(int capacity) {
this.elements = (E[]) new Object[capacity];
top = -1;
}
// 公共方法:入栈
public void push(E item) {
if (top < elements.length - 1) {
top++;
elements[top] = item;
} else {
throw new StackOverflowError("Stack is full");
}
}
// 公共方法:出栈并返回顶部元素
public E pop() {
if (!isEmpty()) {
E item = elements[top];
top--;
return item;
} else {
throw new EmptyStackException("Stack is empty");
}
}
// 公共方法:查看但不移除顶部元素
public E peek() {
if (!isEmpty()) {
return elements[top];
} else {
throw new EmptyStackException("Stack is empty");
}
}
// 公共方法:检查栈是否为空
public boolean isEmpty() {
return top == -1;
}
// 其他必要的辅助方法...
}
```
阅读全文
相关推荐
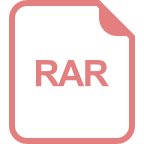
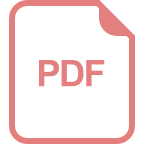
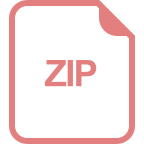
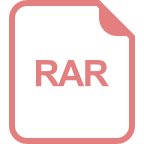
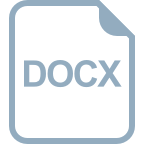
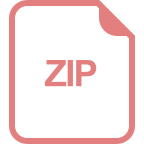
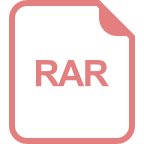
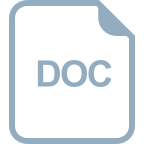
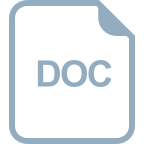
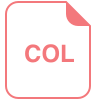
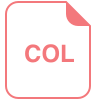
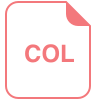
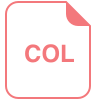
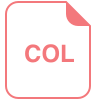



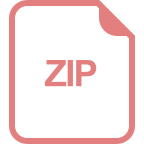