python的resize函数如果比原数组大
时间: 2024-11-10 14:25:28 浏览: 11
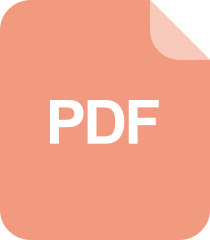
python cv2.resize函数high和width注意事项说明

在Python中,通常涉及到的是NumPy库中的`resize()`函数,它主要用于改变数组的形状(大小),但并不直接改变元素值。如果你想要将数组扩大到比原来大的尺寸,有几种情况:
1. 如果新维度的大小大于原数组对应位置,那么原有数据会被填充。默认情况下,`resize()`会用0填充新元素,除非提供了一个填充值。
```python
import numpy as np
arr = np.array([1, 2, 3])
new_shape = (5, 6) # 新的形状比原数组大
resized_arr = np.resize(arr, new_shape)
print(resized_arr)
```
这将输出一个全零的新数组,形状为(5, 6),因为原数组只有3个元素。
2. 对于一维数组,你可以先将其扩展为二维再调整大小,然后恢复为一维。例如:
```python
arr = np.array([1, 2, 3])
new_size = 7
reshaped = arr.reshape((1, -1)) # 将一维扩展为二维
expanded_arr = np.resize(reshaped, (new_size,))
flattened_arr = expanded_arr.flatten() # 返回一维数组
```
在这种情况下,`expand_arr`将包含额外的0值。
阅读全文
相关推荐
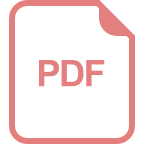
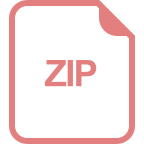

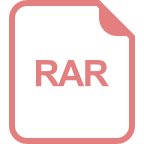
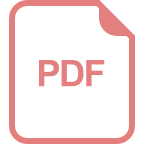
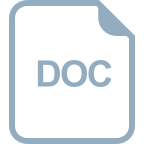
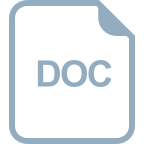
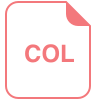
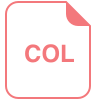
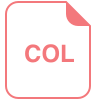








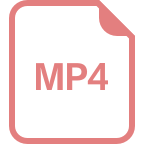