写一个有两个线程的程序,用Thread类来做,第一个线程用来计算2-1000之间的偶数之和及个数,第二个线程用来计算1000-2000之间的偶数之和及个数
时间: 2024-12-20 09:19:43 浏览: 4
为了创建一个有两个线程的Java程序,我们可以使用`Thread`类和`Runnable`接口来实现并发处理。下面是一个简单的示例,我们将创建两个独立的线程,每个线程分别负责计算指定范围内的偶数之和与个数:
```java
public class EvenNumberCalculator implements Runnable {
private int start;
private int end;
private long sum = 0;
private int count = 0;
public EvenNumberCalculator(int start, int end) {
this.start = start;
this.end = end;
}
@Override
public void run() {
for (int i = start; i <= end; i++) {
if (i % 2 == 0) { // 检查是否是偶数
sum += i;
count++;
}
}
System.out.printf("Sum of even numbers in range [%d, %d] is: %d\n", start, end, sum);
System.out.println("Total even numbers in the range are: " + count);
}
public static void main(String[] args) {
int lowRange = 2;
int highRange = 1000;
int secondLowRange = 1000;
int secondHighRange = 2000;
Thread thread1 = new Thread(new EvenNumberCalculator(lowRange, highRange), "Thread1");
Thread thread2 = new Thread(new EvenNumberCalculator(secondLowRange, secondHighRange), "Thread2");
thread1.start();
thread2.start();
try {
// 等待两个线程执行完毕
thread1.join();
thread2.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
```
在这个程序中,我们创建了`EvenNumberCalculator`类并实现了`Runnable`接口。`run()`方法包含了计算过程,并在主线程中启动了两个新的线程,每个线程对应一个特定的偶数范围。
阅读全文
相关推荐
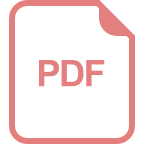
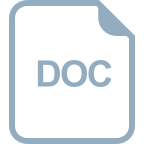
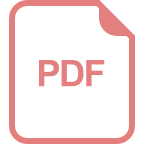















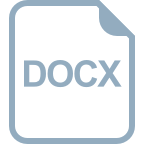