malloc结构体指针指向
时间: 2023-11-22 13:43:35 浏览: 44
malloc函数可以用来动态的分配内存。结构体指针可以通过malloc函数分配适当大小的内存空间,并将其返回给指针。这样,结构体指针就可以指向该内存空间并进行操作。
例如,以下代码分配了一个大小为sizeof(struct student)的内存空间,并将其地址赋值给指向struct student类型的指针变量p:
```
#include <stdio.h>
#include <stdlib.h>
struct student {
char name[20];
int age;
};
int main() {
struct student* p = (struct student*)malloc(sizeof(struct student)); if (p == NULL) {
printf("Failed to allocate memory!\n");
return -1;
}
strcpy(p->name, "Tom");
p->age = 20;
printf("Name: %s, Age: %d\n", p->name, p->age);
free(p);
return 0;
}
```
此代码段中,我们使用malloc函数将一个名为“Tom”的学生结构体变量动态分配到内存中,并将其地址赋值给p。然后我们可以使用p指针变量来访问和修改结构体变量的成员。
相关问题
a结构体指针指向b结构体指针
在C语言中,可以使用结构体指针来指向另一个结构体指针。这样做可以方便地访问和操作结构体成员。
假设有以下两个结构体定义:
```c
typedef struct {
int x;
int y;
} StructA;
typedef struct {
float a;
float b;
StructA* ptr;
} StructB;
```
我们可以创建一个StructA类型的对象a,并将其地址赋值给StructB类型的指针对象b的ptr成员,如下所示:
```c
StructA a;
StructB* b = malloc(sizeof(StructB));
b->ptr = &a;
```
现在,我们可以通过结构体指针b来访问和修改a的成员:
```c
b->ptr->x = 10;
b->ptr->y = 20;
```
结构体指针怎么指向地址
要将结构体指针指向特定的地址,可以使用以下步骤:
1. 声明一个结构体类型。例如,假设你有一个包含姓名和年龄的结构体:
```c
struct Person {
char name[50];
int age;
};
```
2. 声明一个结构体指针变量。例如,使用上述结构体类型声明一个指针变量:
```c
struct Person* personPtr;
```
3. 分配内存给结构体指针。你可以使用 `malloc` 函数来分配内存,为结构体指针指向的地址分配足够的内存空间:
```c
personPtr = (struct Person*)malloc(sizeof(struct Person));
```
4. 使用指针访问结构体成员。通过箭头运算符 `->` 可以使用指针访问结构体成员,例如:
```c
strcpy(personPtr->name, "John");
personPtr->age = 25;
```
这样,你就可以使用结构体指针访问并操作结构体成员了。记得在不需要使用结构体指针时,使用 `free` 函数释放所分配的内存:
```c
free(personPtr);
```
相关推荐
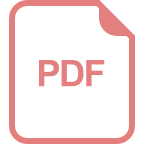
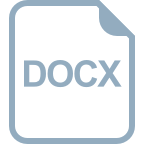
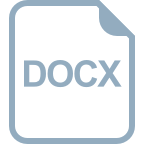
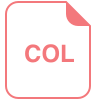
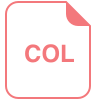
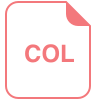
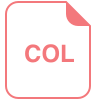
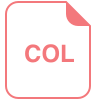







