用c语言写代码 双向链表实现的基本功能 •首/尾增删结点 •获取首/尾部的元素 。清空链表/判空链表/链表大小 •缺省构造/拷贝构造/析构函数 •输出流操作符重载
时间: 2024-05-26 21:07:54 浏览: 19
双向链表是一种常用的数据结构,它可以在O(1)时间内进行插入和删除操作。以下是用C语言实现双向链表的基本功能:
1. 首/尾增删结点
双向链表可以从头部或尾部插入或删除结点。首部插入结点的函数如下:
```
void insert_front(node_t **head, int data) {
node_t *new_node = malloc(sizeof(node_t));
new_node->data = data;
new_node->prev = NULL;
new_node->next = *head;
if (*head != NULL) {
(*head)->prev = new_node;
}
*head = new_node;
}
```
尾部插入结点的函数如下:
```
void insert_back(node_t **head, int data) {
node_t *new_node = malloc(sizeof(node_t));
new_node->data = data;
new_node->prev = NULL;
new_node->next = NULL;
if (*head == NULL) {
*head = new_node;
return;
}
node_t *tail = *head;
while (tail->next != NULL) {
tail = tail->next;
}
tail->next = new_node;
new_node->prev = tail;
}
```
首部删除结点的函数如下:
```
void delete_front(node_t **head) {
if (*head == NULL) {
return;
}
node_t *temp = *head;
*head = (*head)->next;
if (*head != NULL) {
(*head)->prev = NULL;
}
free(temp);
}
```
尾部删除结点的函数如下:
```
void delete_back(node_t **head) {
if (*head == NULL) {
return;
}
node_t *tail = *head;
while (tail->next != NULL) {
tail = tail->next;
}
if (tail == *head) {
*head = NULL;
free(tail);
return;
}
tail->prev->next = NULL;
free(tail);
}
```
2. 获取首/尾部的元素
获取链表头部元素的函数如下:
```
int front(node_t *head) {
if (head == NULL) {
return -1; // or some other value to indicate an error
}
return head->data;
}
```
获取链表尾部元素的函数如下:
```
int back(node_t *head) {
if (head == NULL) {
return -1; // or some other value to indicate an error
}
node_t *tail = head;
while (tail->next != NULL) {
tail = tail->next;
}
return tail->data;
}
```
3. 清空链表/判空链表/链表大小
清空链表的函数如下:
```
void clear(node_t **head) {
while (*head != NULL) {
delete_front(head);
}
}
```
判空链表的函数如下:
```
int is_empty(node_t *head) {
return head == NULL;
}
```
链表大小的函数如下:
```
int size(node_t *head) {
int count = 0;
while (head != NULL) {
count++;
head = head->next;
}
return count;
}
```
4. 缺省构造/拷贝构造/析构函数
缺省构造函数应该初始化链表头为NULL,如下:
```
void init_list(node_t **head) {
*head = NULL;
}
```
拷贝构造函数应该复制整个链表,如下:
```
node_t* copy_list(node_t *src) {
node_t *dest_head = NULL;
while (src != NULL) {
insert_back(&dest_head, src->data);
src = src->next;
}
return dest_head;
}
```
析构函数应该释放整个链表,如下:
```
void destroy_list(node_t **head) {
clear(head);
}
```
5. 输出流操作符重载
输出流操作符重载应该遍历整个链表并输出每个元素,如下:
```
void print_list(node_t *head) {
printf("[");
while (head != NULL) {
printf("%d", head->data);
if (head->next != NULL) {
printf(", ");
}
head = head->next;
}
printf("]\n");
}
```
相关推荐
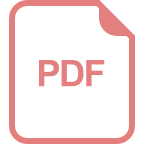
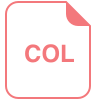
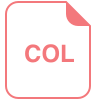
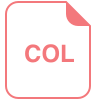
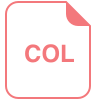
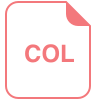









