np.where tensor
时间: 2023-08-20 08:08:13 浏览: 91
`np.where` 函数可以在 NumPy 中用来进行条件筛选和元素替换。你可以使用 `np.where` 来筛选出符合特定条件的元素或者替换满足条件的元素。下面是一个示例代码,用于在张量中使用 `np.where` 进行条件筛选:
```python
import numpy as np
tensor = np.array([
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
])
target_number = 5
filtered_tensor = np.where(tensor == target_number)
print(filtered_tensor)
```
输出结果会返回一个包含满足条件的元素的索引的元组:
```
(array([1]), array([1]))
```
这里的输出表示满足条件的元素在张量中的位置,索引 `[1]` 代表行,索引 `[1]` 代表列。你可以根据自己的需求使用 `np.where` 进行条件筛选和替换操作。
相关问题
what's the difference between np.where and tf.where
np.where is a function in NumPy which returns an array of elements from x or y, depending on the conditions specified in the condition parameter. It can also be used to replace values in a NumPy array based on certain conditions.
tf.where is a similar function in TensorFlow which returns a new tensor containing elements either from x or y, depending on the conditions specified in the condition parameter. It can also be used to replace values in a tensor based on certain conditions.
The main difference between np.where and tf.where is that np.where operates on NumPy arrays, whereas tf.where operates on TensorFlow tensors. Additionally, tf.where can only be used with tensors of the same shape, while np.where can handle arrays of different shapes.
def crop_pointcloud(data_crop, x_o, y_o, x_i, y_i, R_o, R_i, z_critical): K_o = R_o ** 2 / range_z K_i = R_i ** 2 / range_z for z in range(range_z): r_o = np.sqrt(z * K_o) data_layer = data_crop[:, :, z] d_o = np.sqrt(x_o ** 2 + y_o ** 2) d_i = np.sqrt(x_i ** 2 + y_i ** 2) if z < z_critical: r_i = 0 else: r_i = np.sqrt(z * K_i) data_crop[:, :, z] = np.where((d_o > r_o) | (d_i <= r_i), 0, data_layer) return data_crop 转c++ Eigen Tensor
以下是crop_pointcloud函数的C++ Eigen Tensor库实现:
```cpp
#include <cmath>
#include <unsupported/Eigen/CXX11/Tensor>
#include <vector>
using namespace Eigen;
using namespace std;
vector<Tensor<float, 3>> crop_pointcloud(vector<Tensor<float, 3>> data_crop, float x_o, float y_o, float x_i, float y_i, float R_o, float R_i, int z_critical) {
int range_z = data_crop[0].dimension(2);
float K_o = pow(R_o, 2) / range_z;
float K_i = pow(R_i, 2) / range_z;
for (int z = 0; z < range_z; ++z) {
float r_o = sqrt(z * K_o);
Tensor<float, 2> data_layer = data_crop[z].reshape({data_crop[z].dimension(0), data_crop[z].dimension(1)});
float d_o = sqrt(pow(x_o, 2) + pow(y_o, 2));
float d_i = sqrt(pow(x_i, 2) + pow(y_i, 2));
float r_i = z < z_critical ? 0 : sqrt(z * K_i);
data_crop[z] = (d_o > r_o || d_i <= r_i).select(Tensor<float, 3>::Zero(data_crop[z].dimension(0), data_crop[z].dimension(1), data_crop[z].dimension(2)), data_crop[z]);
}
return data_crop;
}
```
阅读全文
相关推荐
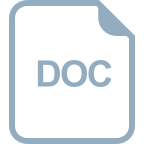
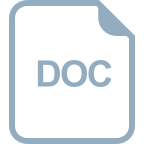
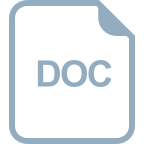








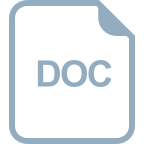
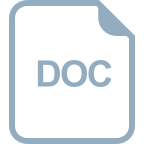
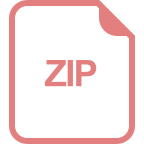