用C语言 :已有邻接表表示的有向图,请编程判断从第u顶点至第v顶点是否有简单路径,若有则印出该路径上的顶点。要求:先描述图的存储结构,并简述算法思路;查找邻接点等图的运算要自己实现。
时间: 2024-04-18 07:28:46 浏览: 54
存储结构:
我们可以使用邻接表来表示有向图。每个顶点都有一个链表,链表中存储了该顶点可达的顶点。
算法思路:
为了判断从顶点 u 到顶点 v 是否有简单路径,我们可以使用深度优先搜索(DFS)算法。从顶点 u 开始,递归地搜索与当前顶点相邻的顶点,并标记已访问的顶点。当找到顶点 v 时,我们可以回溯路径并打印出顶点。
具体实现如下:
```c
#include <stdio.h>
#include <stdbool.h>
#define MAX_VERTICES 100
// 邻接表的链表节点
struct Node {
int vertex;
struct Node* next;
};
// 图的结构体
struct Graph {
struct Node* adjLists[MAX_VERTICES];
bool visited[MAX_VERTICES];
};
// 创建新节点
struct Node* createNode(int v) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->vertex = v;
newNode->next = NULL;
return newNode;
}
// 创建图
struct Graph* createGraph() {
struct Graph* graph = (struct Graph*)malloc(sizeof(struct Graph));
for (int i = 0; i < MAX_VERTICES; i++) {
graph->adjLists[i] = NULL;
graph->visited[i] = false;
}
return graph;
}
// 添加边
void addEdge(struct Graph* graph, int src, int dest) {
// 添加 src -> dest 边
struct Node* newNode = createNode(dest);
newNode->next = graph->adjLists[src];
graph->adjLists[src] = newNode;
}
// DFS遍历
void DFS(struct Graph* graph, int vertex, int dest, bool* found) {
graph->visited[vertex] = true;
if (vertex == dest) {
*found = true;
printf("%d ", vertex);
return;
}
struct Node* adjList = graph->adjLists[vertex];
while (adjList != NULL) {
int connectedVertex = adjList->vertex;
if (!graph->visited[connectedVertex]) {
DFS(graph, connectedVertex, dest, found);
if (*found) {
printf("%d ", vertex);
return;
}
}
adjList = adjList->next;
}
}
// 判断路径
void hasPath(struct Graph* graph, int u, int v) {
bool found = false;
DFS(graph, u, v, &found);
if (found) {
printf("There is a path from %d to %d: ", u, v);
} else {
printf("There is no path from %d to %d\n", u, v);
}
}
int main() {
struct Graph* graph = createGraph();
int u, v;
// 添加边
addEdge(graph, 0, 1);
addEdge(graph, 0, 2);
addEdge(graph, 1, 2);
addEdge(graph, 2, 0);
addEdge(graph, 2, 3);
addEdge(graph, 3, 3);
// 输入起点和终点
printf("Enter the source vertex: ");
scanf("%d", &u);
printf("Enter the destination vertex: ");
scanf("%d", &v);
// 判断路径
hasPath(graph, u, v);
return 0;
}
```
在上述代码中,我们首先定义了邻接表的链表节点结构 `Node` 和图的结构体 `Graph`。然后,我们实现了创建新节点、创建图、添加边、DFS遍历和判断路径的函数。在 `main` 函数中,我们创建了一个有向图并输入起点和终点,然后调用 `hasPath` 函数判断是否存在路径,并输出结果。
注意:上述代码仅为示例,实际使用时需要根据实际情况进行修改。
阅读全文
相关推荐
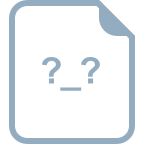
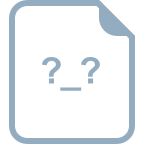

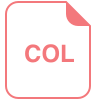













