用c语言实现:输入一组顶点,建立有向图的邻接表。并举例出有效输入
时间: 2024-03-10 08:50:51 浏览: 105
以下是示例代码,使用邻接表表示有向图:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTEX_NUM 100 // 最大顶点数
// 邻接表中的边结构体
typedef struct EdgeNode {
int adjvex; // 邻接顶点编号
struct EdgeNode *next; // 指向下一个邻接点的指针
} EdgeNode;
// 邻接表中的顶点结构体
typedef struct VertexNode {
int vertex; // 顶点编号
EdgeNode *firstedge; // 指向第一个邻接点的指针
} VertexNode;
// 有向图的邻接表结构体
typedef struct {
VertexNode vertexs[MAX_VERTEX_NUM]; // 存储顶点的数组
int vexnum, edgenum; // 顶点数和边数
} GraphAdjList;
// 初始化有向图的邻接表
void InitGraph(GraphAdjList *G, int vexnum) {
G->vexnum = vexnum;
G->edgenum = 0;
// 初始化每个顶点
for (int i = 0; i < vexnum; i++) {
G->vertexs[i].vertex = i;
G->vertexs[i].firstedge = NULL;
}
}
// 添加一条边到有向图的邻接表
void AddEdge(GraphAdjList *G, int tail, int head) {
// 创建一个邻接点
EdgeNode *p = (EdgeNode *)malloc(sizeof(EdgeNode));
p->adjvex = head;
p->next = G->vertexs[tail].firstedge;
G->vertexs[tail].firstedge = p;
G->edgenum++;
}
// 输出有向图的邻接表
void PrintGraph(GraphAdjList G) {
printf("Vertex number: %d, Edge number: %d\n", G.vexnum, G.edgenum);
for (int i = 0; i < G.vexnum; i++) {
printf("Vertex %d: ", G.vertexs[i].vertex);
EdgeNode *p = G.vertexs[i].firstedge;
while (p) {
printf("%d ", p->adjvex);
p = p->next;
}
printf("\n");
}
}
int main() {
int n;
printf("Input number of vertices: ");
scanf("%d", &n);
GraphAdjList G;
InitGraph(&G, n);
printf("Input edges (input -1 -1 to end):\n");
int tail, head;
scanf("%d%d", &tail, &head);
while (tail != -1 && head != -1) {
if (tail < 0 || tail >= n || head < 0 || head >= n) {
printf("Invalid input!\n");
} else {
AddEdge(&G, tail, head);
}
scanf("%d%d", &tail, &head);
}
PrintGraph(G);
return 0;
}
```
输入示例:
```
Input number of vertices: 4
Input edges (input -1 -1 to end):
0 1
1 2
2 3
3 0
-1 -1
```
输出示例:
```
Vertex number: 4, Edge number: 4
Vertex 0: 1
Vertex 1: 2
Vertex 2: 3
Vertex 3: 0
```
阅读全文
相关推荐
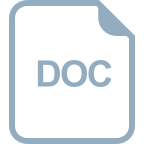
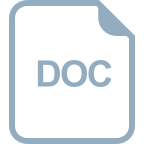
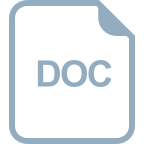






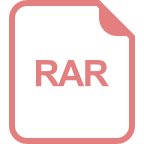
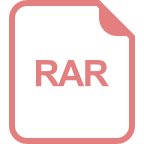
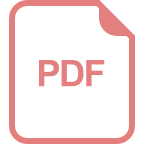
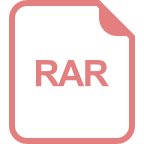
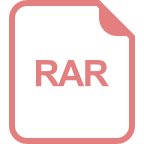
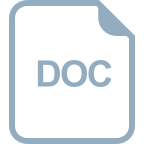
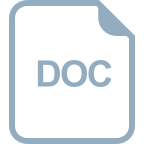

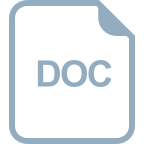