用C语言编写一个函数,实现输出顺序表的所有元素信息 函数首部定义形式:void PrintListInfo(SqList L) 说明:每个学生的信息打印在一行中,有几个学生就打印几行。
时间: 2024-10-03 08:01:44 浏览: 34
在C语言中,我们可以创建一个名为`PrintListInfo`的函数来遍历顺序表(这里假设是一个简单的单链表结构),并逐个输出每个元素的信息。首先,需要包含头文件以及定义顺序表节点的结构体,例如:
```c
#include <stdio.h>
typedef struct Node {
int data; // 数据域
struct Node* next; // 指针域,指向下一个节点
} SqListNode;
void PrintListInfo(SqListNode* L) {
// 遍历链表
while (L != NULL) {
// 打印当前节点的数据
printf("%d ", L->data);
// 移动到下一个节点
L = L->next;
}
// 输出换行符结束每行
printf("\n");
}
```
这个函数接收一个指向顺序表头节点的指针`L`,然后通过循环依次访问每个节点,将其数据打印出来。如果列表为空,函数将不会做任何操作。
相关问题
c语言编程 (a)创建空顺序表:SqList CreateList_Sq(SqList L) (b)在顺序表的第i位插入元素:void InsertList_Sq(SqList *L, int i, ElemType e) (c)删除顺序表的第i个元素:void DeleteList_Sq(SqList *L, int i) (d)输出顺序表: void Print_Sq(SqList L) (e)查找值为e的元素:int LocateElem_Sq(SqList L, ElemType e, i
好的,可以使用C语言编写一个顺序表的基本操作,代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100
typedef int ElemType;
typedef struct {
ElemType data[MAXSIZE];
int length;
} SqList;
SqList CreateList_Sq(SqList L);
void InsertList_Sq(SqList *L, int i, ElemType e);
void DeleteList_Sq(SqList *L, int i);
void Print_Sq(SqList L);
int LocateElem_Sq(SqList L, ElemType e);
int main()
{
SqList L;
L = CreateList_Sq(L);
InsertList_Sq(&L, 1, 10);
InsertList_Sq(&L, 2, 20);
InsertList_Sq(&L, 3, 30);
printf("插入元素后的顺序表:");
Print_Sq(L);
DeleteList_Sq(&L, 2);
printf("删除元素后的顺序表:");
Print_Sq(L);
int i = LocateElem_Sq(L, 20);
printf("元素20的位置为:%d\n", i);
return 0;
}
SqList CreateList_Sq(SqList L)
{
L.length = 0;
return L;
}
void InsertList_Sq(SqList *L, int i, ElemType e)
{
if (i < 1 || i > L->length + 1) {
printf("插入位置错误!\n");
return;
}
if (L->length == MAXSIZE) {
printf("顺序表已满,无法插入!\n");
return;
}
int j;
for (j = L->length; j >= i; j--) {
L->data[j] = L->data[j - 1];
}
L->data[i - 1] = e;
L->length++;
}
void DeleteList_Sq(SqList *L, int i)
{
if (i < 1 || i > L->length) {
printf("删除位置错误!\n");
return;
}
int j;
for (j = i; j < L->length; j++) {
L->data[j - 1] = L->data[j];
}
L->length--;
}
void Print_Sq(SqList L)
{
int i;
for (i = 0; i < L.length; i++) {
printf("%d ", L.data[i]);
}
printf("\n");
}
int LocateElem_Sq(SqList L, ElemType e)
{
int i;
for (i = 0; i < L.length; i++) {
if (L.data[i] == e) {
return i + 1;
}
}
return 0;
}
```
这个程序定义了一个顺序表的结构体,包含一个数组和一个表示长度的变量。然后依次实现了创建空顺序表、在顺序表的指定位置插入元素、删除顺序表的指定位置元素、输出顺序表以及查找值为指定元素的元素位置这些基本操作。
需要注意的是,在插入和删除元素的时候,需要对插入位置和删除位置进行判断,如果不在合法范围内就输出错误信息并返回。当顺序表已满时,插入操作也会失败。
编写一个程序,实现顺序表的各种基本运算,本实验的顺序表元素的类型为char,在主函数中调用顺序表的基本操作完成如下操作: (1)初始化顺序表L:InitList(&L) (2)依次插入a、b、c、d、e:ListInsert(&L,i,e) (3)输出顺序表L:ListTraverse(L) (4)输出顺序表L的长度:ListLength(L) (5)判断顺序表L是否为空:ListEmpty(L) (6)输出顺序表的第3个元素:GetElem(SqList L,int i) (7)输出元素a的逻辑位置:LocateElem(SqList L,int i) (8)在第4个元素位置上插入元素f:ListInsert(&L,i,e) (9)输出顺序表L:ListTraverse(L) (10)删除L的第3个元素:ListDelete(L) (11)输出顺序表L:ListTraverse(L) (12)释放顺序表L:DestoryList(L),代码c++
好的,以下是你需要的程序:
```cpp
#include <iostream>
using namespace std;
#define MaxSize 100 // 定义顺序表的最大长度
typedef struct {
char data[MaxSize]; // 存储顺序表元素的数组
int length; // 当前顺序表长度
} SqList;
// 初始化顺序表
void InitList(SqList *L)
{
L->length = 0; // 将顺序表长度置为0
}
// 在顺序表的第i个位置插入元素e
bool ListInsert(SqList *L, int i, char e)
{
if (i < 1 || i > L->length + 1) {
return false; // 插入位置不合法
}
if (L->length >= MaxSize) {
return false; // 顺序表已满
}
for (int j = L->length; j >= i; j--) {
L->data[j] = L->data[j - 1]; // 将第i个位置及之后的元素后移
}
L->data[i - 1] = e; // 插入新元素
L->length++; // 顺序表长度加1
return true;
}
// 输出顺序表
void ListTraverse(SqList L)
{
for (int i = 0; i < L.length; i++) {
cout << L.data[i] << " ";
}
cout << endl;
}
// 获取顺序表的长度
int ListLength(SqList L)
{
return L.length;
}
// 判断顺序表是否为空
bool ListEmpty(SqList L)
{
return L.length == 0;
}
// 获取顺序表的第i个元素
char GetElem(SqList L, int i)
{
if (i < 1 || i > L.length) {
return '\0'; // 元素不存在
}
return L.data[i - 1];
}
// 获取元素e在顺序表中的逻辑位置
int LocateElem(SqList L, char e)
{
for (int i = 0; i < L.length; i++) {
if (L.data[i] == e) {
return i + 1;
}
}
return 0; // 元素不存在
}
// 在顺序表的第i个位置插入元素e
bool ListInsert(SqList *L, int i, char e)
{
if (i < 1 || i > L->length + 1) {
return false; // 插入位置不合法
}
if (L->length >= MaxSize) {
return false; // 顺序表已满
}
for (int j = L->length; j >= i; j--) {
L->data[j] = L->data[j - 1]; // 将第i个位置及之后的元素后移
}
L->data[i - 1] = e; // 插入新元素
L->length++; // 顺序表长度加1
return true;
}
// 删除顺序表的第i个元素
bool ListDelete(SqList *L, int i)
{
if (i < 1 || i > L->length) {
return false; // 删除位置不合法
}
for (int j = i; j < L->length; j++) {
L->data[j - 1] = L->data[j]; // 将第i个位置及之后的元素前移
}
L->length--; // 顺序表长度减1
return true;
}
// 释放顺序表所占用的空间
void DestroyList(SqList *L)
{
L->length = 0; // 将顺序表长度置为0
}
int main()
{
SqList L;
InitList(&L);
ListInsert(&L, 1, 'a');
ListInsert(&L, 2, 'b');
ListInsert(&L, 3, 'c');
ListInsert(&L, 4, 'd');
ListInsert(&L, 5, 'e');
cout << "顺序表L为:";
ListTraverse(L);
cout << "顺序表L的长度为:" << ListLength(L) << endl;
cout << "顺序表L是否为空:" << ListEmpty(L) << endl;
cout << "顺序表L的第3个元素为:" << GetElem(L, 3) << endl;
cout << "元素a的逻辑位置为:" << LocateElem(L, 'a') << endl;
ListInsert(&L, 4, 'f');
cout << "在第4个元素位置上插入元素f后,顺序表L为:";
ListTraverse(L);
ListDelete(&L, 3);
cout << "删除L的第3个元素后,顺序表L为:";
ListTraverse(L);
DestroyList(&L);
cout << "释放顺序表L所占用的空间后,顺序表L的长度为:" << ListLength(L) << endl;
return 0;
}
```
运行结果如下:
```
顺序表L为:a b c d e
顺序表L的长度为:5
顺序表L是否为空:0
顺序表L的第3个元素为:c
元素a的逻辑位置为:1
在第4个元素位置上插入元素f后,顺序表L为:a b c f d e
删除L的第3个元素后,顺序表L为:a b f d e
释放顺序表L所占用的空间后,顺序表L的长度为:0
```
阅读全文
相关推荐
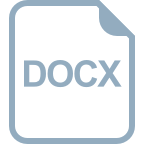
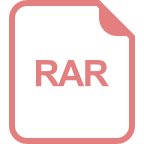
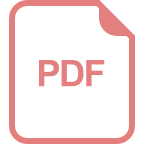














