用C语言解决如下问题存在一个按升序排列的链表,给你这个链表的头节点 head ,请你删除所有重复的元素,使每个元素只出现一次 。返回同样按升序排列的结果链表。
时间: 2024-10-11 09:14:47 浏览: 33
在C语言中,解决这个问题可以采用哈希集合(如`unordered_set`)来辅助操作,同时保持链表的顺序。以下是大致步骤:
1. 定义一个新的单向链表结构,用于存储去重后的结果。
2. 创建一个空的哈希集合(这里假设使用`typedef struct hash_node{int value; bool in_hash; struct hash_node *next;}`作为哈希集合的节点)。
3. 遍历给定的输入链表,对于每一个节点:
a. 如果该节点的值不在哈希集合中(`in_hash == false`),将其添加到结果链表并将值添加到哈希集合中。
b. 否则,表示已遇到重复值,跳过当前节点。
4. 每处理完一个节点,将结果链表的`next`指针指向下一个未处理的节点。
5. 遍历结束后,最后的结果链表就是去重后的链表。
下面是一个伪代码示例:
```c
struct Node* removeDuplicates(struct Node* head) {
if (head == NULL || head->next == NULL) return head;
// 创建新的链表头
struct Node* new_head = NULL;
struct hash_node* hash_set = NULL;
struct Node* current = head;
while (current != NULL) {
if (!hash_set->find(current->value)) { // 如果值不在哈希集合中
// 创建新节点并添加到结果链表
struct Node* newNode = malloc(sizeof(struct Node));
newNode->value = current->value;
newNode->next = new_head;
new_head = newNode;
// 添加到哈希集合
struct hash_node* newHashNode = malloc(sizeof(hash_node));
newHashNode->value = current->value;
newHashNode->in_hash = true;
newHashNode->next = hash_set;
hash_set = newHashNode;
}
current = current->next;
}
return new_head;
}
```
阅读全文
相关推荐
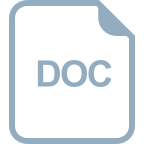
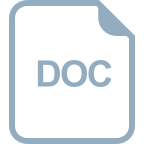
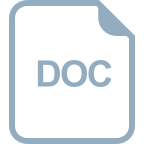
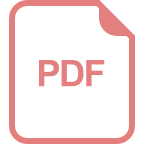














