根据一个数组绘制直方图
时间: 2024-12-02 18:17:48 浏览: 1
在Python中,我们可以使用matplotlib和numpy库一起创建直方图,基于一个给定的数组。下面是一个基本的例子:
首先,假设我们有一个名为`data`的一维数组:
```python
import numpy as np
import matplotlib.pyplot as plt
# 示例数组
data = np.random.randn(1000)
# 绘制直方图
plt.hist(data, bins=20, edgecolor='black', alpha=0.7) # bins设置分割区间数
# 显示x轴和y轴标签
plt.xlabel('随机值')
plt.ylabel('频率')
# 显示图表标题
plt.title('随机数据分布直方图')
# 展示图形
plt.show()
```
在这个例子中:
- `np.random.randn(1000)`生成了一个包含1000个随机标准正态分布数值的数组。
- `hist`函数用于绘制直方图,第一个参数是要分析的数组,`bins=20`指定了我们将数据分为20个区间。
- `edgecolor`设定了边界颜色,`alpha`设置了透明度,使其更美观。
- `xlabel`和`ylabel`设置了坐标轴标签,`title`设置了图表的标题。
运行这段代码后,你就得到了一个表示`data`数组数据分布的直方图。
相关问题
如何根据一个数组绘制频次直方图
根据一个数组绘制频次直方图,你可以使用Python的NumPy库配合Matplotlib库。以下是基本步骤:
1. 导入所需的库:
```python
import numpy as np
import matplotlib.pyplot as plt
```
2. 准备数组,这可以是你的原始数据:
```python
data = [1, 2, 3, 3, 3, 4, 5, 6, 7, 8, 8, 9]
```
3. 使用`numpy.histogram`函数计算频率分布,并获取bin的边界和频率(count):
```python
hist, bins = np.histogram(data, bins='auto')
```
`bins='auto'`会自动选择合适的bin数量以适应数据,如果想指定bin的数量,可以用`bins=range(min(data), max(data) + 1)`。
4. 创建直方图:
```python
plt.bar(bins[:-1], hist, width=(bins[1] - bins[0])) # bar宽度设定为bin间距
```
`bins[:-1]`用于获取bin的中心点,因为直方图通常是基于bin中心绘制的。
5. 添加标签、标题和显示图形:
```python
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.title('Histogram of Data')
plt.show()
```
完整的代码示例:
```python
import numpy as np
import matplotlib.pyplot as plt
data = [1, 2, 3, 3, 3, 4, 5, 6, 7, 8, 8, 9]
hist, bins = np.histogram(data, bins='auto')
plt.bar(bins[:-1], hist, width=(bins[1] - bins[0]))
plt.xlabel('Value')
plt.ylabel('Frequency')
plt.title('Histogram of Data')
plt.show()
```
运行此代码后,你应该就能看到根据给定数组绘制出的频次直方图了。
matlab根据一个数组绘制频次统计直方图
你可以使用MATLAB中的`histogram()`函数来绘制频次统计直方图。下面是一个示例代码:
```matlab
% 生成一组随机数据
data = randn(1000,1);
% 绘制直方图
histogram(data);
```
在这个示例中,我们生成了一个包含1000个随机数的一维数组`data`,然后使用`histogram()`函数绘制了它的频次统计直方图。你可以根据自己的数据替换这个示例代码中的`data`数组,以绘制自己的频次统计直方图。
阅读全文
相关推荐
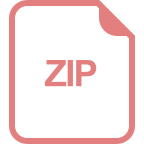
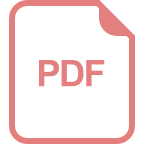
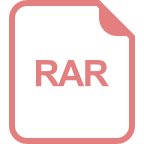
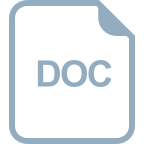


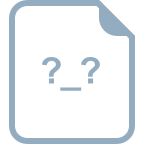
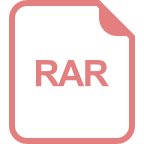
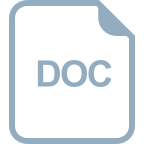
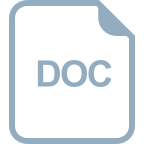






