vue3执行函数传入屏幕对象获取想x,y
时间: 2024-09-18 21:09:01 浏览: 38
在Vue3中,如果你想在组件内部执行一个函数并传递当前元素(比如屏幕对象,通常是指某个DOM元素)的位置信息,如x和y坐标,你可以这样做:
首先,在组件的模板或计算属性中,假设有一个`ref`用于绑定屏幕元素,例如一个`<div>`:
```html
<div ref="screenElement"></div>
```
然后,在JavaScript部分,可以创建一个自定义事件或者在函数中访问这个`ref`的值,并从中提取x和y坐标:
```javascript
<script setup lang="ts">
import { ref } from 'vue';
const screenElement = ref(null); // 或者直接使用v-ref
function getPosition() {
if (screenElement.value) {
const position = screenElement.value.getBoundingClientRect();
const x = position.left;
const y = position.top;
// 然后你可以返回一个对象或者直接操作x和y变量
return { x, y };
}
}
</script>
```
在这个例子中,`getPosition`函数会在屏幕元素存在时获取其位置。如果元素未渲染或者已被移除,`getBoundingClientRect()`会返回`null`。
相关问题
如何调试 canvasToTempFilePath 方法: 确保 canvasToTempFilePath 方法中的回调函数正确执行,并返回预期的结果对象。 并检查是否有任何异步操作未能完成或抛出异常
为了调试 `canvasToTempFilePath` 方法并确保其回调函数正确执行且返回预期结果,可以按照以下步骤进行:
### 1. 添加日志记录
在关键位置添加 `console.log` 语句,以跟踪方法的执行流程和变量状态。
### 2. 检查回调函数
确保回调函数(如 `success` 和 `fail`)被正确调用,并传递正确的参数。
### 3. 捕获异步错误
使用 `try...catch` 块捕获可能的异步错误。
### 具体步骤如下:
#### 修改 `canvasToTempFilePath` 方法
```javascript
methods: {
canvasToTempFilePath(param = {}) {
console.log('canvasToTempFilePath method called with param:', param);
const { success = () => {}, fail = (err) => console.error(err) } = param;
try {
const isEmpty = this.isEmpty();
console.log('isEmpty:', isEmpty);
// #ifdef APP-NVUE
this.$refs.webview.evalJS(`save()`);
const stopURLWatch = this.$watch('toDataURL', (v, n) => {
console.log('toDataURL changed from', n, 'to', v);
if (v && v !== n) {
if (param.pathType == 'url') {
base64ToPath(v).then(res => {
console.log('Base64 to path conversion successful:', res);
success({ tempFilePath: res, isEmpty });
}).catch(err => {
console.error('Base64 to path conversion failed:', err);
fail(err);
});
} else {
console.log('Returning base64 data URL:', v);
success({ tempFilePath: v, isEmpty });
}
this.toDataURL = '';
stopURLWatch && stopURLWatch();
}
});
const { fileType, quality } = param;
const rsave = JSON.parse(this.rsave);
rsave.n++;
rsave.fileType = fileType;
rsave.quality = quality;
this.rsave = JSON.stringify(rsave);
// #endif
// #ifndef APP-VUE || APP-NVUE
const { canvas } = this.signature.canvas.get('el');
const { backgroundColor, landscape, boundingBox } = this;
let width = this.signature.canvas.get('width');
let height = this.signature.canvas.get('height');
let x = 0;
let y = 0;
const canvasToTempFilePath = (image) => {
const context = uni.createCanvasContext('offscreen', this);
context.save();
context.setTransform(1, 0, 0, 1, 0, 0);
if (landscape) {
context.translate(0, width);
context.rotate(-Math.PI / 2);
}
if (backgroundColor) {
context.fillStyle = backgroundColor;
context.fillRect(0, 0, width, height);
}
context.drawImage(image, 0, 0, width, height);
context.draw(false, () => {
toDataURL('offscreen', this, param).then((res) => {
const size = Math.max(width, height);
context.restore();
context.clearRect(0, 0, size, size);
console.log('Generated temp file path:', res);
success({ tempFilePath: res, isEmpty });
}).catch(err => {
console.error('Failed to generate temp file path:', err);
fail(err);
});
});
};
const next = () => {
// #ifndef MP-WEIXIN
const param = { x, y, width, height, canvas };
// #endif
// #ifdef MP-WEIXIN
const param = { x, y, width, height, canvas: this.useCanvas2d ? canvas : null };
// #endif
toDataURL(this.canvasId, this, param).then(canvasToTempFilePath).catch(fail);
};
// PC端小程序获取不到 ImageData 数据,长度为0
if (boundingBox && !isPC) {
this.signature.getContentBoundingBox(async res => {
this.offscreenWidth = width = res.width;
this.offscreenHeight = height = res.height;
x = res.startX;
y = res.startY;
await sleep(100);
next();
});
} else {
next();
}
// #endif
} catch (error) {
console.error('Error in canvasToTempFilePath:', error);
fail(error);
}
},
}
```
### 4. 测试方法
调用 `canvasToTempFilePath` 方法并传入适当的参数,观察控制台输出的日志,确保每个步骤都按预期执行。
### 5. 验证结果
检查回调函数 `success` 是否被正确调用,并验证返回的对象是否包含预期的 `tempFilePath` 和 `isEmpty` 属性。
通过以上步骤,你可以更有效地调试 `canvasToTempFilePath` 方法,确保其正常工作并处理可能出现的异常情况。
如何在Vue项目中实现数字的动态竖向翻牌效果,包括数字的渲染、位置变换以及平滑过渡的动画处理?请结合CSS的writing-mode、transform和transition属性详细描述实现过程。
在Vue项目中实现数字的动态竖向翻牌效果,首先需要理解CSS中`writing-mode: vertical-lr`属性的作用,它使得数字可以竖向排列,然后通过`transform: translate()`来控制数字的移动。对于动画效果的实现,则需要使用`transition`属性为数字的变换过程添加平滑的过渡效果。具体步骤如下:
参考资源链接:[Vue实现竖向数字动态翻牌:CSS与动画核心技术](https://wenku.csdn.net/doc/6412b703be7fbd1778d48c76?spm=1055.2569.3001.10343)
1. **数字布局**:在Vue模板中,使用`v-for`指令渲染数字列表,并为每个数字分配一个包含适当样式类的容器,这些类会根据是否为数字还是分隔符(如逗号)来应用不同的样式。
2. **CSS设置**:定义一个`.number-item`或类似的类,利用`writing-mode: vertical-lr`来使数字竖向排列。然后,设置`transform: translate()`以确保数字能够通过改变Y轴坐标来在竖直方向上切换位置。同时,为这些变化添加`transition`属性来实现动画效果。
3. **JavaScript逻辑**:在Vue组件的JavaScript部分,根据需要显示的数字序列初始化一个数组,这个数组会被用来通过`v-for`指令动态渲染数字。使用`mounted`生命周期钩子来设置动画的触发时机,例如通过`setTimeout`延迟执行一个函数,该函数将数字数组传入`setNumberTransform`方法来触发翻牌效果。
4. **动画触发与控制**:编写`setNumberTransform`方法,该方法需要计算每个数字的新位置,并更新数组状态以反映数字的变化。同时,该方法会触发CSS中定义的`transition`动画,使得数字翻转看起来平滑且自然。
通过上述步骤,结合CSS和JavaScript的方法,可以在Vue项目中实现一个具有动画效果的数字翻牌组件,使得数字的显示更加生动和吸引用户注意。需要注意的是,虽然这里没有提供`setNumberTransform`方法的具体实现,但根据上述步骤的描述,你可以根据项目需求进一步开发和优化这一功能。
为了更全面地掌握和应用这些技术点,建议深入学习《Vue实现竖向数字动态翻牌:CSS与动画核心技术》这篇文章。文章详细解析了数字翻牌效果的实现原理和关键技术点,可以帮助你构建出更加完善和复杂的动画效果。
参考资源链接:[Vue实现竖向数字动态翻牌:CSS与动画核心技术](https://wenku.csdn.net/doc/6412b703be7fbd1778d48c76?spm=1055.2569.3001.10343)
阅读全文
相关推荐
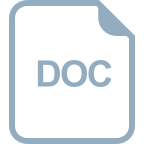
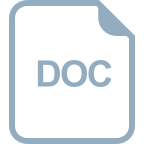
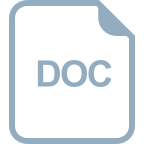
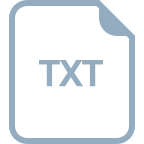
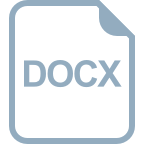
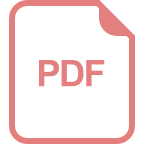
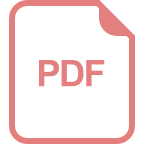
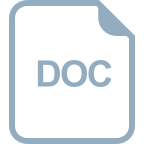
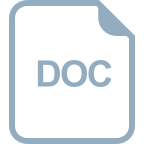
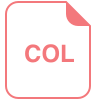
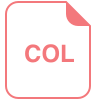
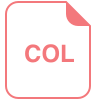
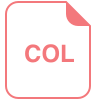
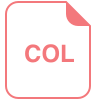
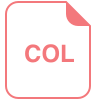
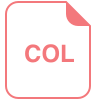
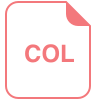