推箱子c 程序代码
时间: 2024-09-26 07:03:14 浏览: 21
推箱子(Push Box Game)是一种经典的计算机科学问题,通常用作算法和数据结构的教学示例。它涉及到在一个二维网格上移动物体,目标通常是将特定的盒子推到指定的位置。这里是一个简单的推箱子程序的伪代码描述,基于递归回溯算法:
```python
# 定义一个方格类
class Grid:
def __init__(self, grid):
self.grid = grid
self.box_positions = []
# 检查当前位置是否可以放置盒子
def can_place_box(self, row, col):
return self.grid[row][col] == '.'
# 递归函数,尝试把盒子推到目标位置
def push_to_target(self, box_pos, target_pos, current_pos):
if self.solve(box_pos, target_pos, current_pos): # 解决成功
return True
else:
for move in [(0, -1), (0, 1), (-1, 0), (1, 0)]: # 四个方向尝试移动
new_box_pos = (box_pos[0] + move[0], box_pos[1] + move[1])
if self.can_move(new_box_pos, current_pos): # 可以移动
if self.push_to_target(new_box_pos, target_pos, current_pos):
return True # 成功后回溯
return False
# 检查能否从当前位置移动到另一个位置
def can_move(self, pos1, pos2):
# ... (检查路径合法性等)
# 主函数
def main():
grid_str = "..." # 用字符串表示网格
grid = Grid(grid_str)
box_pos = (0, 0) # 盒子初始位置
target_pos = (3, 3) # 目标位置
if grid.push_to_target(box_pos, target_pos, None): # 开始游戏
print("Success!")
else:
print("Failed.")
if __name__ == "__main__":
main()
```
这只是一个基本框架,实际代码会更复杂,包括路径搜索、阻碍物处理和边界条件检查等。如果你对C语言感兴趣,你可以将其转换成C代码,但核心逻辑是一致的。
阅读全文
相关推荐
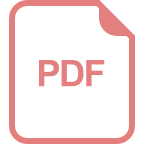
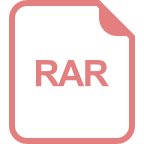
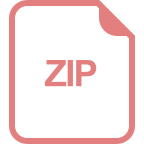





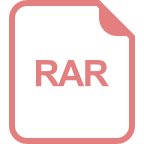
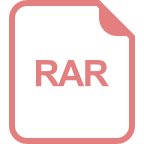

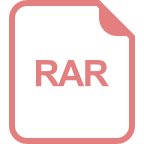
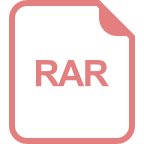
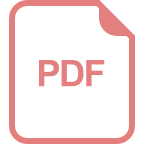
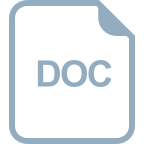
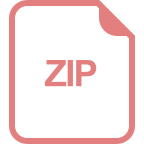

