pyton中缀表达式转换为后缀表达式。
时间: 2023-10-08 18:13:22 浏览: 83
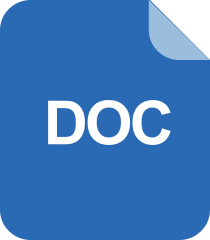
【精品】中缀表达式转换为后缀表达式的一般算法.doc
在Python中将中缀表达式转换为后缀表达式的一种常见方法是使用栈。
具体步骤如下:
1. 创建一个空栈和一个空列表。
2. 从左到右遍历中缀表达式的每个字符。
3. 如果当前字符是数字,将其添加到列表的末尾。
4. 如果当前字符是左括号,将其压入栈中。
5. 如果当前字符是右括号,则将栈中所有操作符弹出并添加到列表中,直到遇到左括号为止。然后将左括号弹出但不添加到列表中。
6. 如果当前字符是操作符,将其压入栈中。但在此之前,需要先将栈中所有优先级大于或等于该操作符的操作符弹出并添加到列表中。
7. 当表达式的所有字符都已遍历完毕时,将栈中所有操作符弹出并添加到列表中。
8. 最终列表中的元素就是后缀表达式。
以下是一个示例代码:
```
def infix_to_postfix(expression):
precedence = {'+': 1, '-': 1, '*': 2, '/': 2, '^': 3}
stack = []
postfix = []
for char in expression:
if char.isdigit():
postfix.append(char)
elif char == '(':
stack.append(char)
elif char == ')':
while stack[-1] != '(':
postfix.append(stack.pop())
stack.pop()
else:
while stack and stack[-1] != '(' and precedence[char] <= precedence.get(stack[-1], 0):
postfix.append(stack.pop())
stack.append(char)
while stack:
postfix.append(stack.pop())
return postfix
```
使用示例:
```
expression = '3+4*2/(1-5)^2'
postfix = infix_to_postfix(expression)
print(postfix) # ['3', '4', '2', '*', '1', '5', '-', '2', '^', '/', '+']
```
阅读全文
相关推荐
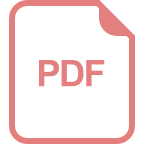
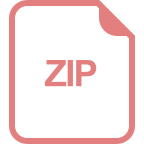
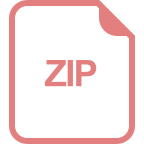
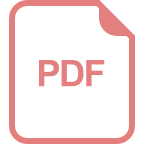









