用restful api设计并实现shipping and transportation服务,给我一段代码
时间: 2023-07-15 14:11:07 浏览: 159
这里提供一个简单的示例代码,实现了一个基于RESTful API的货物运输服务。
```python
from flask import Flask, jsonify, request
app = Flask(__name__)
# Mock database for demonstration purposes
shipments = [
{"id": 1, "name": "Shipment 1", "origin": "New York", "destination": "Los Angeles"},
{"id": 2, "name": "Shipment 2", "origin": "Chicago", "destination": "Boston"}
]
# Get all shipments
@app.route('/shipments', methods=['GET'])
def get_shipments():
return jsonify(shipments)
# Get a shipment by ID
@app.route('/shipments/<int:id>', methods=['GET'])
def get_shipment(id):
shipment = next((shipment for shipment in shipments if shipment["id"] == id), None)
if shipment:
return jsonify(shipment)
else:
return jsonify({"error": "Shipment not found"}), 404
# Create a new shipment
@app.route('/shipments', methods=['POST'])
def create_shipment():
shipment = request.get_json()
shipment["id"] = max(shipments, key=lambda x:x["id"])["id"] + 1 # auto-increment ID
shipments.append(shipment)
return jsonify(shipment)
# Update a shipment by ID
@app.route('/shipments/<int:id>', methods=['PUT'])
def update_shipment(id):
shipment = next((shipment for shipment in shipments if shipment["id"] == id), None)
if shipment:
updated_shipment = request.get_json()
shipment.update(updated_shipment)
return jsonify(shipment)
else:
return jsonify({"error": "Shipment not found"}), 404
# Delete a shipment by ID
@app.route('/shipments/<int:id>', methods=['DELETE'])
def delete_shipment(id):
shipment = next((shipment for shipment in shipments if shipment["id"] == id), None)
if shipment:
shipments.remove(shipment)
return jsonify({"message": "Shipment deleted"})
else:
return jsonify({"error": "Shipment not found"}), 404
if __name__ == '__main__':
app.run(debug=True)
```
这个示例代码使用了Python的Flask框架,定义了五个不同的路由,分别对应获取所有货运、按ID获取单个货运、创建新货运、更新现有货运和删除现有货运。每个路由都提供了RESTful API所需的HTTP方法,并使用JSON格式作为数据传输格式。
阅读全文
相关推荐


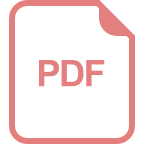
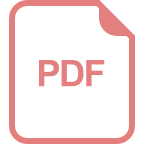
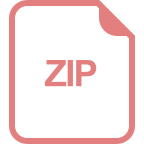
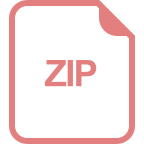
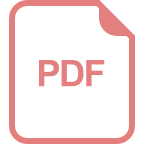
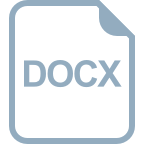

