c语言 求方程式ax2+bx+c=0的代码
时间: 2023-12-21 12:03:42 浏览: 147
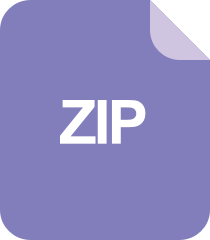
c代码-求ax平方+bx+c+=0
以下是求解一元二次方程ax^2 + bx + c = 0的C语言代码:
```c
#include <stdio.h>
#include <math.h>
int main() {
double a, b, c, delta, x1, x2;
printf("请输入一元二次方程的系数(a, b, c):\n");
scanf("%lf %lf %lf",&a, &b, &c);
delta = b*b - 4*a*c;
if(delta > 0) {
x1 = (-b + sqrt(delta)) / (2*a);
x2 = (-b - sqrt(delta)) / (2*a);
printf("方程有两个不相等的实根:x1 = %.2lf, x2 = %.2lf\n", x1, x2);
} else if(delta == 0) {
x1 = x2 = -b / (2*a);
printf("方程有两个相等的实根:x1 = x2 = %.2lf\n", x1);
} else {
printf("方程没有实根\n");
}
return 0;
}
```
注:sqrt()函数需要在math.h库中引入。
阅读全文
相关推荐
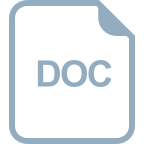
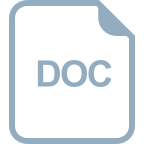















