class Employee { public: int id; // 员工编号 char name[20]; // 员工姓名 char position[20]; // 员工岗位厂长部门主管车间主任普通员工 int k; // 等级 int num; // 绩效得分/计件个数(根据员工具体类别而定) int fulldays, actualdays, overdays; // 应到天数实到天数加班天数 float bonus; // 奖励工资 };
时间: 2023-08-06 08:07:52 浏览: 45
这段代码定义了一个名为 Employee 的类,它包含了员工的编号、姓名、岗位、等级、绩效得分、工作日数、加班天数和奖励工资等信息。基于这个类,你可以创建员工对象,并对员工的属性进行操作和管理。
以下是一个重新编写的 Employee 类的示例代码:
```
class Employee {
public:
Employee(int id, const char* name, const char* position, int k, int num, int fulldays, int actualdays, int overdays, float bonus)
: id(id), k(k), num(num), fulldays(fulldays), actualdays(actualdays), overdays(overdays), bonus(bonus) {
strcpy(this->name, name);
strcpy(this->position, position);
}
int getId() const {
return id;
}
const char* getName() const {
return name;
}
const char* getPosition() const {
return position;
}
int getLevel() const {
return k;
}
int getPerformance() const {
return num;
}
int getFullDays() const {
return fulldays;
}
int getActualDays() const {
return actualdays;
}
int getOverDays() const {
return overdays;
}
float getBonus() const {
return bonus;
}
void setName(const char* name) {
strcpy(this->name, name);
}
void setPosition(const char* position) {
strcpy(this->position, position);
}
void setLevel(int k) {
this->k = k;
}
void setPerformance(int num) {
this->num = num;
}
void setFullDays(int fulldays) {
this->fulldays = fulldays;
}
void setActualDays(int actualdays) {
this->actualdays = actualdays;
}
void setOverDays(int overdays) {
this->overdays = overdays;
}
void setBonus(float bonus) {
this->bonus = bonus;
}
private:
int id;
char name[20];
char position[20];
int k;
int num;
int fulldays;
int actualdays;
int overdays;
float bonus;
};
```
这个示例代码重新定义了 Employee 类,并增加了访问器和设置器,以便对类的私有成员进行访问和修改。同时,构造函数也被重新定义,以便在创建对象时初始化类的私有成员。
相关推荐
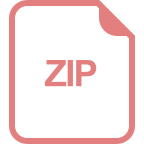
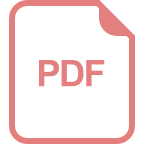
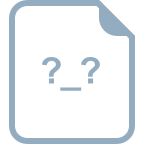








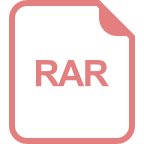
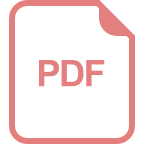
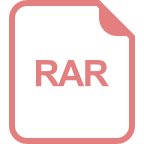
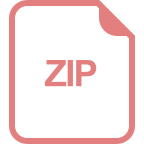
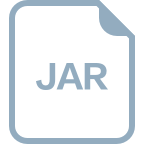