单应性矩阵计算 Python
时间: 2023-11-29 21:44:46 浏览: 237
单应性矩阵是计算机视觉中的一个重要概念,它是指在两个平面之间存在一种映射关系,可以将一个平面上的点映射到另一个平面上。在计算机视觉中,单应性矩阵通常用于图像配准、图像拼接、三维重建等领域。
在Python中,可以使用OpenCV库来计算单应性矩阵。具体步骤如下:
1.导入必要的库
```python
import cv2
import numpy as np
```
2.读取图像
```python
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
```
3.提取特征点
```python
sift = cv2.xfeatures2d.SIFT_create()
kp1, des1 = sift.detectAndCompute(img1, None)
kp2, des2 = sift.detectAndCompute(img2, None)
```
4.匹配特征点
```python
bf = cv2.BFMatcher()
matches = bf.knnMatch(des1, des2, k=2)
good = []
for m, n in matches:
if m.distance < 0.75 * n.distance:
good.append(m)
```
5.计算单应性矩阵
```python
src_pts = np.float32([kp1[m.queryIdx].pt for m in good]).reshape(-1, 1, 2)
dst_pts = np.float32([kp2[m.trainIdx].pt for m in good]).reshape(-1, 1, 2)
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
```
其中,src_pts和dst_pts分别是两幅图像中匹配的特征点坐标,M是计算得到的单应性矩阵。
6.输出结果
```python
print(M)
```
以上就是使用Python计算单应性矩阵的步骤。如果需要更详细的解释,可以参考OpenCV官方文档。
阅读全文
相关推荐



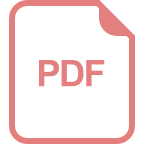
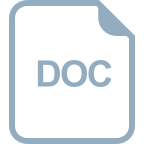

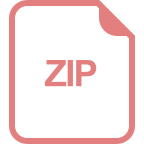
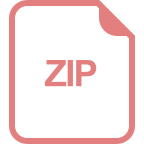
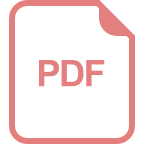
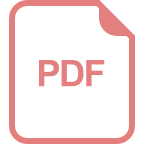
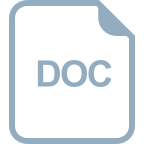




