用字符数组作函数参数编程实现如下功能:在字符串中删除与某字符相同的字符。 **提示信息: "input a string:" "input a character:" **输入格式要求:"%s" **输出格式要求:"results:%s\n" 程序运行示例1如下: input a string:hello,world! input a character:o results:hell,wrld!
时间: 2023-04-29 20:06:43 浏览: 111
这道题目要求我们在一个字符数组中删除指定的字符,并输出结果。输入格式为 "input a string:" 和 "input a character:",使用的格式化要求为 "%s",输出结果要求使用格式化要求 "results:%s\n"。以下是样例代码:
```
def delete_char(s, c):
s = list(s)
for i in range(len(s)):
if s[i] == c:
s[i] = ""
return ''.join(s)
if __name__ == '__main__':
s = input("input a string:")
c = input("input a character:")
result = delete_char(s, c)
print("results:%s\n" % result)
```
示例输出:
```
input a string:hello,world!
input a character:o
results:hell,wrld!
```
相关问题
用字符数组作函数参数编程实现如下功能,在字符事中删除与某字符相同的字符。 **提示信息。 "Input a string: " "Input a character: "*输入格式要求:"%s" 输出格式要求:“Results:%sNn
好的,以下是实现该功能的 C++ 代码:
```c++
#include <iostream>
#include <cstring>
using namespace std;
void deleteChar(char str[], char ch) {
int len = strlen(str);
int j = 0;
for (int i = 0; i < len; i++) {
if (str[i] != ch) {
str[j++] = str[i];
}
}
str[j] = '\0';
}
int main() {
char str[100];
char ch;
cout << "Input a string: ";
cin >> str;
cout << "Input a character: ";
cin >> ch;
deleteChar(str, ch);
cout << "Results: " << str << endl;
return 0;
}
```
程序的主要思路是遍历字符串,将不与给定字符相同的字符依次存入原字符串中,最后在字符串末尾加上一个空字符 '\0',表示字符串结束。
用字符指针作函数参数编程实现如下功能:在字符串中删除与某字符相同的字符。 **提示信息: "Input a string:" "Input a character:" **输入格式要求:"%s" **输出格式要求:"Results:%s\n" 程序运行示例1如下: Input a string:hello,world! Input a character:o Results:hell,wrld!
下面是代码实现:
```c
#include <stdio.h>
void delete_char(char *str, char ch) {
int i, j;
for (i = 0, j = 0; str[i] != '\0'; i++) {
if (str[i] != ch) {
str[j] = str[i];
j++;
}
}
str[j] = '\0';
}
int main() {
char str[100], ch;
printf("Input a string:");
scanf("%s", str);
printf("Input a character:");
scanf(" %c", &ch);
delete_char(str, ch);
printf("Results:%s\n", str);
return 0;
}
```
首先在 `main` 函数中读入字符串和待删除的字符,然后调用 `delete_char` 函数进行字符删除操作。在 `delete_char` 函数中,使用双指针法对字符串进行遍历和修改,当当前字符不等于待删除的字符时,将其复制到新的位置,最终再在末尾插入字符串结尾符号 `\0` 以结束字符串。
注意在读入字符时要注意加上一个空格,以避免读入上一次输入时的回车符。
阅读全文
相关推荐
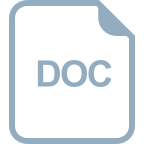
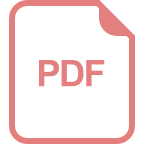













