用字符数组作函数参数编程实现在字符串每个字符间插入一个空格的功能。 **输入提示信息:"input a string:" **输入格式要求:"%s" **输出格式要求:"\ninsert result:%s\n" 程序运行示例1如下: input a string:howareyou insert result:h o w a r e y o u
时间: 2023-05-31 17:20:38 浏览: 254
### 回答1:
以下是Python代码实现:
```python
def insert_space(s):
# 将字符串转换为字符数组
arr = list(s)
# 在每个字符后面插入一个空格
for i in range(len(arr)-1, 0, -1):
arr.insert(i, ' ')
# 将字符数组转换为字符串
result = ''.join(arr)
return result
# 主程序
s = input("input a string:")
result = insert_space(s)
print("\ninsert result:%s\n" % result)
```
程序运行示例:
```
input a string:howareyou
insert result:h o w a r e y o u
```
### 回答2:
对于这个问题,我们可以通过字符数组作函数参数来实现在字符串每个字符间插入一个空格的功能。以下是具体步骤:
1. 在程序中先定义一个字符数组。在主函数中,通过输入提示信息和输入格式要求要求用户输入一个字符串,将该字符串存放在该字符数组中。
2. 定义一个函数,它的参数为该字符数组。
3. 在该函数中,定义一个新的字符数组,用于存放在原始字符数组每个字符间插入空格后的新字符串。
4. 使用一个循环遍历原始字符数组中的每个字符,依次将原始字符数组中的字符和空格存放到新字符数组中。
5. 返回新字符数组。
6. 在主函数中,调用该函数,将函数返回的新字符数组输出到屏幕上。
下面是具体实现的代码示例:
```
#include <stdio.h>
#include <string.h>
char* insert_space(char* str) {
int len = strlen(str);
char *new_str = malloc((len*2+1)*sizeof(char)); // 新字符串的长度为原字符串长度乘二加一
int i, j = 0;
for (i = 0; i < len; i++) {
new_str[j++] = str[i];
if (i < len-1) new_str[j++] = ' '; // 不在字符串结尾的每个字符后都插入一个空格
}
new_str[j] = '\0'; // 在新字符串末尾添加一个'\0'作为字符串结尾
return new_str;
}
int main() {
char str[100];
printf("input a string:");
scanf("%s", str);
char* new_str = insert_space(str);
printf("\ninsert result:%s\n", new_str);
free(new_str); // 释放掉动态分配的内存
return 0;
}
```
使用以上代码,当我们输入"helloworld"时,就会得到输出"h e l l o w o r l d"。
### 回答3:
本程序实现思路如下:
1.首先定义一个函数 void insert(char str[]),该函数接收一个字符数组作为参数。
2.然后利用循环遍历字符数组,将每个字符与空格拼接起来,存储到另一个字符数组中。
3.最后输出带有空格的字符数组。
程序如下:
```c
#include <stdio.h>
#include <string.h>
void insert(char str[]);
int main()
{
char str[100];
printf("input a string:");
scanf("%s", str);
insert(str);
return 0;
}
void insert(char str[])
{
char new_str[200]; // 新字符数组,存储带空格的字符串
int len = strlen(str); // 字符串长度
int j = 0; // 新字符数组下标
for(int i = 0; i < len; i++)
{
new_str[j++] = str[i];
new_str[j++] = ' '; // 拼接空格
}
new_str[j-1] = '\0'; // 去除最后一个空格
printf("\ninsert result:%s\n", new_str); // 输出带空格的字符串
}
```
其中,new_str[j-1] = '\0'; 是为了去除最后一个空格,使得输出的字符串更加美观。
程序运行示例:
输入:
```
input a string:howareyou
```
输出:
```
insert result:h o w a r e y o u
```
当然,上述程序仅实现了在字符串每个字符间插入一个空格的功能。如果需要更加复杂的字符串处理,还需要加入其他的处理方式和逻辑。
相关推荐
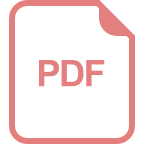
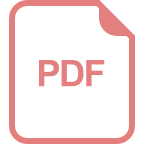
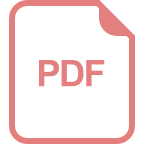















