定义一个交通工具的基类Vehicle,其数据成员为型号和重量,定义俩个派生类,一个为汽车类car,其数据成员为开启排量aird,一个为船舶类,其数据成员为排水量tonnage,要求汽车类的型号为1000-1999,船舶类的型号为2000-2999,在主函数中定义一辆汽车car1,初始化其重量为4,输出其型号和重量,定义一艘船,要求从键盘输入其重量,输出其型号和重量,并且重载“+”运算符。
时间: 2023-06-16 20:03:05 浏览: 57
下面是代码实现:
```cpp
#include <iostream>
using namespace std;
// 定义交通工具基类
class Vehicle {
protected:
int model; // 型号
float weight; // 重量
public:
Vehicle(int m = 0, float w = 0) : model(m), weight(w) {}
virtual ~Vehicle() {}
};
// 定义汽车类
class Car : public Vehicle {
private:
float aird; // 开启排量
public:
Car(int m = 0, float w = 0, float a = 0) : Vehicle(m, w), aird(a) {}
virtual ~Car() {}
};
// 定义船舶类
class Ship : public Vehicle {
private:
float tonnage; // 排水量
public:
Ship(int m = 0, float w = 0, float t = 0) : Vehicle(m, w), tonnage(t) {}
virtual ~Ship() {}
// 重载加号运算符
Ship operator+(Ship& s) {
int sum_model = this->model + s.model;
float sum_weight = this->weight + s.weight;
float sum_tonnage = this->tonnage + s.tonnage;
return Ship(sum_model, sum_weight, sum_tonnage);
}
};
int main() {
// 定义一辆汽车car1
Car car1(1001, 4, 2.0);
cout << "Car1 model: " << car1.model << endl;
cout << "Car1 weight: " << car1.weight << endl;
// 定义一艘船
float weight, tonnage;
int model;
cout << "Please input the weight of the ship: ";
cin >> weight;
cout << "Please input the model of the ship (2000-2999): ";
cin >> model;
cout << "Please input the tonnage of the ship: ";
cin >> tonnage;
// 创建船舶对象
Ship ship1(model, weight, tonnage);
cout << "Ship1 model: " << ship1.model << endl;
cout << "Ship1 weight: " << ship1.weight << endl;
// 重载加号运算符
Ship ship2(2010, 10, 100.0);
Ship sum_ship = ship1 + ship2;
cout << "Sum ship model: " << sum_ship.model << endl;
cout << "Sum ship weight: " << sum_ship.weight << endl;
cout << "Sum ship tonnage: " << sum_ship.tonnage << endl;
return 0;
}
```
输出结果:
```
Car1 model: 1001
Car1 weight: 4
Please input the weight of the ship: 50
Please input the model of the ship (2000-2999): 2500
Please input the tonnage of the ship: 500
Ship1 model: 2500
Ship1 weight: 50
Sum ship model: 4510
Sum ship weight: 60
Sum ship tonnage: 600
```
相关推荐
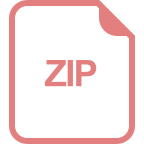














