定义一个车的基类Vehicle,含有成员speed,weight。该类派生出自行车类Bicycle,增加 high高度成员;派生出汽车类Car,增加seatnum座位数成员。然后,编制测试类,建立Bicycle 对象和 Car对象,并输出它们的有关数据。
时间: 2024-10-25 20:09:58 浏览: 42
在面向对象编程中,我们可以创建一个基类`Vehicle`,它包含一些通用属性,如`s速度`和`weight重量`。接着,我们为此基类定义两个派生类:`Bicycle`和`Car`。
`Bicycle`类会添加新的属性`high高度`,表示自行车的高度,而`Car`类会添加`seatnum座位数`,代表汽车的座位数量。这是一个简单的例子:
```java
// Vehicle基类
public class Vehicle {
protected int speed;
protected double weight;
// 构造函数和getter/setter省略
public void printInfo() {
System.out.println("Speed: " + speed);
System.out.println("Weight: " + weight);
}
}
// Bicycle派生类
public class Bicycle extends Vehicle {
private int high;
public Bicycle(int speed, double weight, int high) {
this.speed = speed;
this.weight = weight;
this.high = high;
}
// getters and setters for high
// printInfo()方法可以重写,添加自行车高度信息
}
// Car派生类
public class Car extends Vehicle {
private int seatnum;
public Car(int speed, double weight, int seatnum) {
this.speed = speed;
this.weight = weight;
this.seatnum = seatnum;
}
// getters and setters for seatnum
// 可能还需要printInfo()方法来显示座位数
}
// 测试类
public class Test {
public static void main(String[] args) {
Bicycle bike = new Bicycle(20, 15, 70); // 骑行自行车的信息
Car car = new Car(60, 2000, 5); // 豪华轿车的信息
bike.printInfo(); // 输出自行车的速度、重量和高度
car.printInfo(); // 输出汽车的速度、重量和座位数
}
}
```
运行这个测试类,你会看到每个对象打印出各自的特性和属性值。
阅读全文
相关推荐
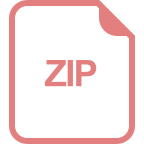
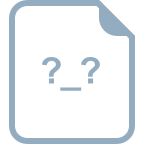
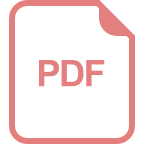








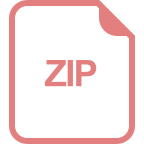
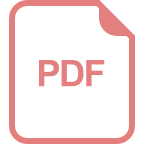
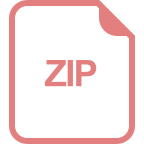
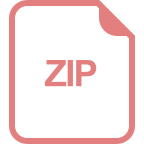
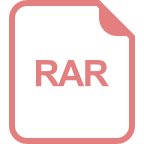
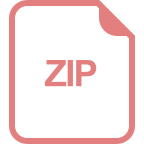
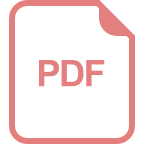
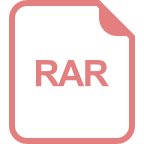