定义一个车基类Vehicle,含私有成员speed,weight。派生出自行车类Bicycle,增加height(高度)成员;汽车类Car,增加seatNum(座位数)成员。实现Vehicle, Bicycle和Car三个类,使主函数能顺利执行并得到预期结果。 #include <iostream> using namespace std; …… int main() { Vehicle v(100,500); v.run(); Car car(120,400,6); car.run(); Bicycle bicycle(30,10,26); bicycle.run(); Vehicle *vp; vp=&car; vp->run(); vp=&bicycle; vp->run(); return 0; } 得到的结果是: Weight 500, speed 100 Weight 400, speed 120 seatNum: 6 Weight 10, speed 30 height: 26 Weight 400, speed 120 seatNum: 6 Weight 10, speed 30 height: 26
时间: 2024-01-17 19:05:25 浏览: 41
好的,这是一个基础的面向对象编程问题,下面是实现代码:
```
#include <iostream>
using namespace std;
class Vehicle {
private:
int speed;
int weight;
public:
Vehicle(int s, int w) : speed(s), weight(w) {}
virtual void run() {
cout << "Weight " << weight << ", speed " << speed << endl;
}
};
class Bicycle : public Vehicle {
private:
int height;
public:
Bicycle(int s, int w, int h) : Vehicle(s, w), height(h) {}
void run() {
Vehicle::run();
cout << "height: " << height << endl;
}
};
class Car : public Vehicle {
private:
int seatNum;
public:
Car(int s, int w, int num) : Vehicle(s, w), seatNum(num) {}
void run() {
Vehicle::run();
cout << "seatNum: " << seatNum << endl;
}
};
int main() {
Vehicle v(100, 500);
v.run();
Car car(120, 400, 6);
car.run();
Bicycle bicycle(30, 10, 26);
bicycle.run();
Vehicle *vp;
vp = &car;
vp->run();
vp = &bicycle;
vp->run();
return 0;
}
```
在这个实现中,我们首先定义了一个基类Vehicle,包含私有成员speed和weight,并实现了虚函数run(),用于输出基类对象的属性。然后我们分别派生出自行车类Bicycle和汽车类Car,增加各自的成员变量height和seatNum,并覆盖了基类的run()函数,用于输出各自的属性。最后在主函数中创建了各种对象并输出其属性,包括使用基类指针调用派生类的虚函数。
相关推荐
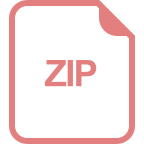
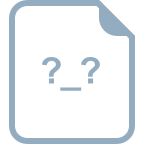
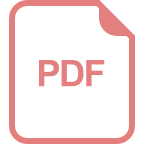
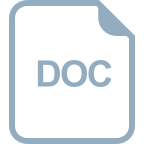
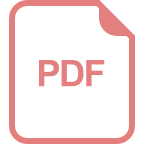
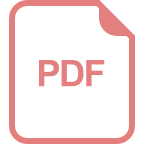
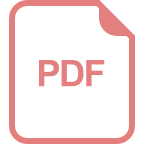
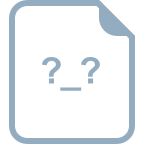
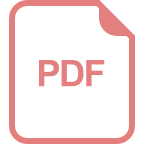
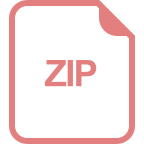
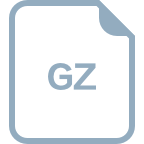
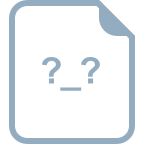
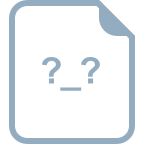
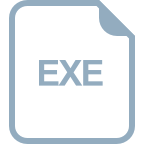
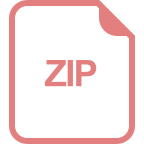
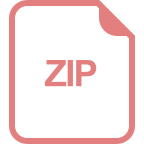
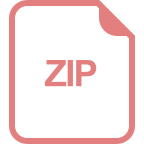