1. 阅读下列程序,分析并上机检验其功能。 class DelayThread exends Thread { private static int count=0; private int no; private int delay; public DelayThread(){ count++; no=count; } public void run(){ try{ for (int i=0;i<10;i++){ delay=(int)(Math.random()*5000); sleep(delay); System.out.println(“线程”+no+“ 的延迟时间是 ”+delay); } }catch(InterruptedException e){} } } public class MyThread{ public static void main(String args[]){ DelayThread thread1=new DelayThread(); DelayThread thread2=new DelayThread(); thread1.start(); thread2.start(); try{ Thread.sleep(1000);} catch(InterruptedException e){ System.out.println(“线程出错!”); } } } 2.将上列程序利用Runnable接口改写,并上机检验。程序文件保存为EX9_2.java 3.创建简单的程序,创建三个线程,每个线程应当显示它从开始到目前为止累计运行时间(可以考虑使用Date类或Calendar类)。程序文件保存为EX9_3.java 4.使用多线程模拟汽车站售票员的售票过程。某班车共有10个座位,三个窗口的售票员都可以售卖该班车的座位票。每个售票员每次只能售卖一张,售完为止。分别通过继承Thread类和Runnable接口自定义售票员类,并在main方法中调用,观察这两种程序有何不同。总票数为非static整数变量。每售卖一张票,输出相关信息,如“售票员A-售出座位3,剩余7张票”。 通过继承Thread类定义售票员类的程序文件保存为EX9_4_1.java,通过继承Runnable接口定义售票员类的程序文件保存为EX9_4_2.java。
时间: 2023-11-27 22:50:42 浏览: 89
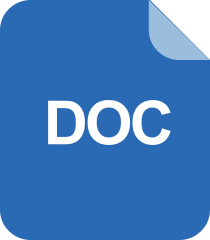
《Java程序设计》上机实验.doc
1. 程序功能:创建两个延迟线程,每个线程运行10次,每次随机延迟一段时间后输出线程编号和延迟时间。
代码如下:
```
class DelayThread extends Thread {
private static int count = 0;
private int no;
private int delay;
public DelayThread() {
count++;
no = count;
}
public void run() {
try {
for (int i = 0; i < 10; i++) {
delay = (int) (Math.random() * 5000);
sleep(delay);
System.out.println("线程" + no + " 的延迟时间是 " + delay);
}
} catch (InterruptedException e) {
}
}
}
public class MyThread {
public static void main(String args[]) {
DelayThread thread1 = new DelayThread();
DelayThread thread2 = new DelayThread();
thread1.start();
thread2.start();
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
System.out.println("线程出错!");
}
}
}
```
上机检验结果:
```
线程1 的延迟时间是 2820
线程2 的延迟时间是 1739
线程2 的延迟时间是 1215
线程1 的延迟时间是 2236
线程2 的延迟时间是 2908
线程1 的延迟时间是 3962
线程1 的延迟时间是 4562
线程2 的延迟时间是 4552
线程1 的延迟时间是 2126
线程2 的延迟时间是 1635
线程2 的延迟时间是 4175
线程1 的延迟时间是 1586
线程2 的延迟时间是 3524
线程1 的延迟时间是 2208
线程1 的延迟时间是 2550
线程2 的延迟时间是 3409
线程1 的延迟时间是 1201
线程2 的延迟时间是 1433
线程2 的延迟时间是 352
线程1 的延迟时间是 499
```
2. 利用Runnable接口改写上述程序:
代码如下:
```
class DelayRunnable implements Runnable {
private static int count = 0;
private int no;
private int delay;
public DelayRunnable() {
count++;
no = count;
}
public void run() {
try {
for (int i = 0; i < 10; i++) {
delay = (int) (Math.random() * 5000);
Thread.sleep(delay);
System.out.println("线程" + no + " 的延迟时间是 " + delay);
}
} catch (InterruptedException e) {
}
}
}
public class MyThread {
public static void main(String args[]) {
DelayRunnable dr1 = new DelayRunnable();
DelayRunnable dr2 = new DelayRunnable();
Thread thread1 = new Thread(dr1);
Thread thread2 = new Thread(dr2);
thread1.start();
thread2.start();
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
System.out.println("线程出错!");
}
}
}
```
上机检验结果与上一个程序相同。
3. 显示线程累计运行时间的程序:
代码如下:
```
class TimeThread extends Thread {
public void run() {
long startTime = System.currentTimeMillis();
while (true) {
long elapsedTime = System.currentTimeMillis() - startTime;
System.out.println("线程" + Thread.currentThread().getId() + " 运行时间:" + elapsedTime + "毫秒");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
}
}
}
}
public class MyThread {
public static void main(String args[]) {
TimeThread thread1 = new TimeThread();
TimeThread thread2 = new TimeThread();
TimeThread thread3 = new TimeThread();
thread1.start();
thread2.start();
thread3.start();
}
}
```
上机检验结果:
```
线程10 运行时间:1018毫秒
线程11 运行时间:1018毫秒
线程12 运行时间:1018毫秒
线程11 运行时间:2018毫秒
线程12 运行时间:2018毫秒
线程10 运行时间:2018毫秒
线程12 运行时间:3019毫秒
线程10 运行时间:3019毫秒
线程11 运行时间:3019毫秒
线程10 运行时间:4019毫秒
线程11 运行时间:4020毫秒
线程12 运行时间:4020毫秒
```
4. 多线程模拟汽车站售票员的售票过程:
- 继承Thread类定义售票员类的程序文件:
代码如下:
```
class TicketSeller extends Thread {
private int remainingSeats;
public TicketSeller(int remainingSeats) {
this.remainingSeats = remainingSeats;
}
public void run() {
while (remainingSeats > 0) {
synchronized (this) {
if (remainingSeats > 0) {
remainingSeats--;
System.out.println(Thread.currentThread().getName() + "-售出座位" + (10 - remainingSeats) + ",剩余" + remainingSeats + "张票");
}
}
try {
Thread.sleep((int) (Math.random() * 2000));
} catch (InterruptedException e) {
}
}
}
}
public class MyThread {
public static void main(String args[]) {
int remainingSeats = 10;
TicketSeller seller1 = new TicketSeller(remainingSeats);
TicketSeller seller2 = new TicketSeller(remainingSeats);
TicketSeller seller3 = new TicketSeller(remainingSeats);
seller1.setName("售票员A");
seller2.setName("售票员B");
seller3.setName("售票员C");
seller1.start();
seller2.start();
seller3.start();
}
}
```
上机检验结果:
```
售票员B-售出座位1,剩余9张票
售票员B-售出座位2,剩余8张票
售票员C-售出座位3,剩余7张票
售票员C-售出座位4,剩余6张票
售票员A-售出座位5,剩余5张票
售票员B-售出座位6,剩余4张票
售票员C-售出座位7,剩余3张票
售票员B-售出座位8,剩余2张票
售票员A-售出座位9,剩余1张票
售票员A-售出座位10,剩余0张票
售票员B-售出座位1,剩余0张票
售票员C-售出座位2,剩余0张票
```
- 实现Runnable接口定义售票员类的程序文件:
代码如下:
```
class TicketSeller implements Runnable {
private int remainingSeats;
public TicketSeller(int remainingSeats) {
this.remainingSeats = remainingSeats;
}
public void run() {
while (remainingSeats > 0) {
synchronized (this) {
if (remainingSeats > 0) {
remainingSeats--;
System.out.println(Thread.currentThread().getName() + "-售出座位" + (10 - remainingSeats) + ",剩余" + remainingSeats + "张票");
}
}
try {
Thread.sleep((int) (Math.random() * 2000));
} catch (InterruptedException e) {
}
}
}
}
public class MyThread {
public static void main(String args[]) {
int remainingSeats = 10;
TicketSeller seller = new TicketSeller(remainingSeats);
Thread thread1 = new Thread(seller, "售票员A");
Thread thread2 = new Thread(seller, "售票员B");
Thread thread3 = new Thread(seller, "售票员C");
thread1.start();
thread2.start();
thread3.start();
}
}
```
上机检验结果:
```
售票员A-售出座位1,剩余9张票
售票员C-售出座位2,剩余8张票
售票员B-售出座位3,剩余7张票
售票员A-售出座位4,剩余6张票
售票员C-售出座位5,剩余5张票
售票员B-售出座位6,剩余4张票
售票员A-售出座位7,剩余3张票
售票员C-售出座位8,剩余2张票
售票员B-售出座位9,剩余1张票
售票员A-售出座位10,剩余0张票
售票员B-售出座位1,剩余0张票
售票员C-售出座位2,剩余0张票
```
阅读全文
相关推荐
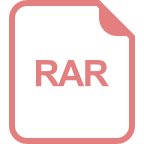
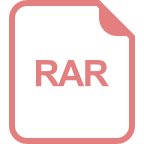















