c++哈希表不用类和结构体实现怎么代码实现
时间: 2023-05-25 19:05:32 浏览: 105
哈希表可以用数组和链表实现,具体实现方式如下:
1. 定义一个数组,数组的长度为哈希表中键值对的最大数量(一般为质数),数组中每个元素的初始值为 NULL。
2. 定义一个哈希函数,将键值通过该函数转换成数组下标。
3. 实现插入函数。首先使用哈希函数获取键对应的数组下标,然后在该下标对应的链表中查找键是否已经存在,如果存在,则更新其对应的值;如果不存在,则在链表头插入新节点,节点的值为键值对。
4. 实现查找函数。同样使用哈希函数获取键对应的数组下标,然后在该下标对应的链表中查找键是否存在,如果存在,则返回其对应的值;如果不存在,则返回空值。
5. 实现删除函数。同样使用哈希函数获取键对应的数组下标,然后在该下标对应的链表中查找键是否存在,如果存在,则删除该节点,否则不做任何处理。
下面是一个实现哈希表的示例代码(使用 C 语言实现):
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 10007
#define KEY_SIZE 128
struct Node {
char key[KEY_SIZE];
char value[KEY_SIZE];
struct Node* next;
};
struct Node* hashtable[MAX_SIZE] = { NULL };
int hash(char* key) {
int sum = 0;
for (int i = 0; i < strlen(key); i++) {
sum += key[i];
}
return sum % MAX_SIZE;
}
void insert(char* key, char* value) {
int index = hash(key);
struct Node* p = hashtable[index];
while (p != NULL) {
if (strcmp(p->key, key) == 0) {
strcpy(p->value, value);
return;
}
p = p->next;
}
struct Node* node = (struct Node*)malloc(sizeof(struct Node));
strcpy(node->key, key);
strcpy(node->value, value);
node->next = hashtable[index];
hashtable[index] = node;
}
char* find(char* key) {
int index = hash(key);
struct Node* p = hashtable[index];
while (p != NULL) {
if (strcmp(p->key, key) == 0) {
return p->value;
}
p = p->next;
}
return NULL;
}
void remove_key(char* key) {
int index = hash(key);
struct Node* p = hashtable[index];
if (p == NULL) {
return;
}
if (strcmp(p->key, key) == 0) {
hashtable[index] = p->next;
free(p);
return;
}
while (p->next != NULL) {
if (strcmp(p->next->key, key) == 0) {
struct Node* node = p->next;
p->next = node->next;
free(node);
return;
}
p = p->next;
}
}
int main() {
insert("hello", "world");
insert("foo", "bar");
printf("%s\n", find("hello")); // output: world
printf("%s\n", find("foo")); // output: bar
remove_key("foo");
printf("%s\n", find("foo")); // output: (null)
return 0;
}
```
阅读全文
相关推荐

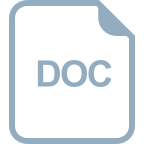


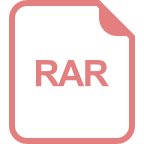
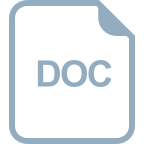
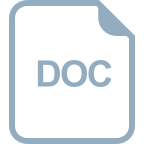
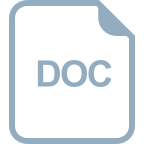
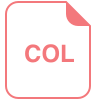
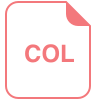




