unity json加密解密
时间: 2024-08-11 10:01:40 浏览: 55
在Unity游戏开发中,JSON(JavaScript Object Notation)是一种常用的轻量级数据交换格式,它将数据序列化为易于阅读和编写的形式。然而,如果你需要对敏感信息如用户账号密码等进行加密后再存储到JSON文件中,可以采取以下步骤:
1. 加密:使用Unity内置的System.Security.Cryptography命名空间提供的算法(如AES、RSA等),对JSON字符串进行加密。例如,你可以创建一个自定义的Encryptor类,封装加密操作。
```csharp
using System.Text.Json;
using System.Security.Cryptography;
public class Encryptor {
public string Encrypt(string jsonData, string encryptionKey) {
byte[] encryptedBytes = AESEncryption(jsonData, encryptionKey);
return Convert.ToBase64String(encryptedBytes);
}
private byte[] AESEncryption(string input, string key) {
// 实现AES加密逻辑
}
}
```
2. 存储:将加密后的字符串保存到JSON文件中,替换原始明文数据。
3. 解密:读取JSON文件时,先从文件获取加密数据,然后通过相同的密钥和同样的加密算法进行解密。
```csharp
public class Decryptor {
public string Decrypt(string encryptedJson, string decryptionKey) {
byte[] decryptedBytes = AESDecryption(Convert.FromBase64String(encryptedJson), decryptionKey);
return Encoding.UTF8.GetString(decryptedBytes);
}
private byte[] AESDecryption(byte[] encryptedBytes, string key) {
// 实现AES解密逻辑
}
}
```
注意:在实际项目中,你应该妥善管理密钥的安全,避免将其硬编码到代码中。可以考虑使用安全的方式存储和传输密钥,比如环境变量或安全的服务。
相关推荐
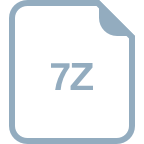
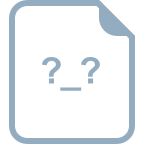
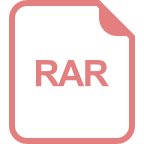














