在 Unity 中保存和载入游戏
时间: 2024-05-07 17:17:55 浏览: 95
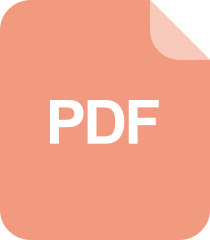
Unity实现游戏存档框架
在 Unity 中,可以通过使用PlayerPrefs和Serialization来保存和载入游戏。
PlayerPrefs是一个简单的键值对存储,可以用来存储玩家的偏好设置、游戏进度等信息。使用PlayerPrefs保存数据时,需要指定一个唯一的键名和对应的值,例如:
```
PlayerPrefs.SetInt("Level", 3);
PlayerPrefs.SetString("PlayerName", "Tom");
```
使用Serialization可以将游戏对象和场景保存到文件中,再在需要时将它们载入并还原。使用Serialization保存数据时,需要定义一个Serializable类来存储数据,例如:
```
[System.Serializable]
public class GameData {
public int level;
public string playerName;
}
GameData data = new GameData();
data.level = 3;
data.playerName = "Tom";
BinaryFormatter bf = new BinaryFormatter();
FileStream file = File.Create(Application.persistentDataPath + "/game.dat");
bf.Serialize(file, data);
file.Close();
```
在这个示例中,我们定义了一个GameData类来存储游戏数据,然后使用BinaryFormatter将GameData对象序列化为二进制数据并保存到文件中。
要载入保存的数据,可以使用以下代码:
```
if (File.Exists(Application.persistentDataPath + "/game.dat")) {
BinaryFormatter bf = new BinaryFormatter();
FileStream file = File.Open(Application.persistentDataPath + "/game.dat", FileMode.Open);
GameData data = (GameData)bf.Deserialize(file);
file.Close();
// 使用data中的数据还原游戏状态
}
```
在这个示例中,我们首先检查保存数据的文件是否存在,如果存在则使用BinaryFormatter从文件中读取数据并将其反序列化为GameData对象,然后使用GameData中的数据还原游戏状态。
阅读全文
相关推荐
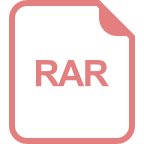
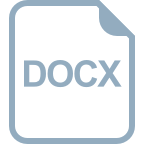
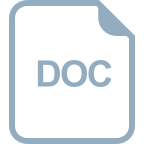
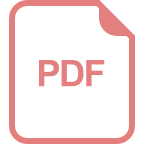
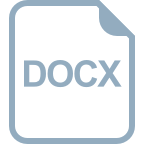
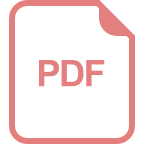
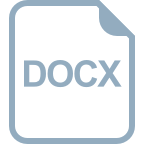
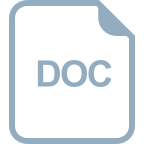
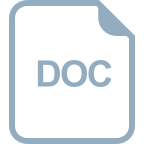
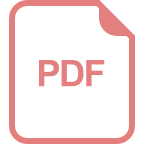
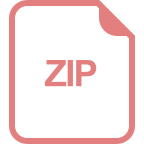
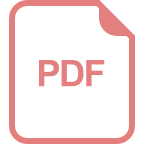
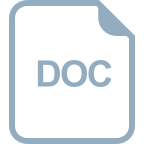
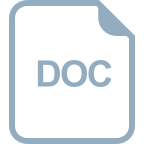
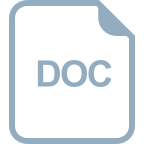
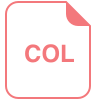
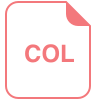