python 用Bagging和Random Forest组合分类 代码
时间: 2024-02-09 07:08:27 浏览: 20
以下是使用Python中scikit-learn库实现Bagging和Random Forest组合分类算法的代码示例:
```python
# 导入需要的库和数据集
from sklearn.datasets import load_iris
from sklearn.ensemble import BaggingClassifier, RandomForestClassifier
from sklearn.model_selection import train_test_split
# 加载数据集
iris = load_iris()
X = iris.data
y = iris.target
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# 构建Bagging分类器
bagging = BaggingClassifier(base_estimator=RandomForestClassifier(),
n_estimators=10, max_samples=0.8, max_features=0.8)
# 训练Bagging分类器
bagging.fit(X_train, y_train)
# 预测测试集结果
bagging_y_pred = bagging.predict(X_test)
# 输出Bagging分类器的准确率
print("Bagging Classifier Accuracy:", bagging.score(X_test, y_test))
# 构建Random Forest分类器
random_forest = RandomForestClassifier(n_estimators=10, max_depth=5, max_features=0.8)
# 训练Random Forest分类器
random_forest.fit(X_train, y_train)
# 预测测试集结果
random_forest_y_pred = random_forest.predict(X_test)
# 输出Random Forest分类器的准确率
print("Random Forest Classifier Accuracy:", random_forest.score(X_test, y_test))
```
在上面的代码中,我们使用`load_iris()`函数加载鸢尾花数据集,并将其划分为训练集和测试集。然后,我们使用`BaggingClassifier`和`RandomForestClassifier`分别构建Bagging分类器和Random Forest分类器,并通过`fit()`方法训练它们。最后,我们可以使用`score()`方法计算分类器的准确率,并输出结果。
相关推荐
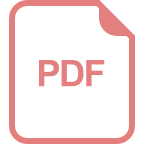
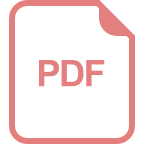
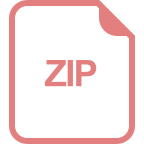
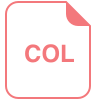













