data: () => ({ atom: atom, itemsPerPage: namingUtils.getNameByCd('paginationUnitDefault', '0'), pageCount: 0, page: 1, }), methods: { updateRowsPerPage(number) { this.itemsPerPage = number; this.page = 1; this.$emit('updatePaginationInfo', { page: this.page, itemsPerPage: this.itemsPerPage, }); }, doPage() { this.$emit('updatePaginationInfo', { page: this.page, itemsPerPage: this.itemsPerPage, }); }, init() { this.page = this.pPage; this.itemsPerPage = this.pItemsPerPage; }, }, computed: { pageNumFirst: function () { let num = 0; if (this.itemsPerPage == -1) { num = 1; } else { num = 1 + (this.page - 1) * this.itemsPerPage; } if (num > this.pItemsLength) { num = this.pItemsLength; } return num; }, pageNumLast: function () { let num = 0; if (this.itemsPerPage == -1) { num = this.pItemsLength; } else { num = this.page * this.itemsPerPage; } if (num > this.pItemsLength) { num = this.pItemsLength; } return num; }, }, created: function () { this.init(); }, watch: { pPage() { this.page = this.pPage; }, pItemsPerPage() { this.itemsPerPage = this.pItemsPerPage; }, pItemsLength() { this.pageCount = Math.ceil(this.pItemsLength / this.itemsPerPage); }, }, };解释代码
时间: 2024-02-14 16:31:22 浏览: 27
这段代码是一个Vue组件的定义,它包含了一些属性、方法、计算属性、生命周期钩子和监听器。我将逐一解释每个部分的作用:
1. data: () => ({...}): 这是组件的数据对象,包含了组件需要响应式地跟踪的属性。在这个例子中,有四个属性:atom、itemsPerPage、page和pageCount。
2. methods: {...}: 这是组件的方法对象,包含了组件需要执行的一些操作。在这个例子中,有三个方法:updateRowsPerPage、doPage和init。
3. computed: {...}: 这是计算属性对象,用于动态地计算和追踪属性的值。在这个例子中,有两个计算属性:pageNumFirst和pageNumLast。
4. created: function (): 这是一个生命周期钩子函数,在组件被创建时被调用。在这个例子中,它调用了init方法。
5. watch: {...}: 这是一个监听器对象,用于监听指定属性的变化并做出相应的响应。在这个例子中,监听了pPage、pItemsPerPage和pItemsLength这三个属性的变化。
总体来说,这个组件用于实现分页功能,并提供了更新每页条目数、执行分页操作和初始化的方法。计算属性则用于动态计算页码的起始和结束位置。
相关问题
vue3分页组件@update:current-page
下面是一个基于 Vue 3 的分页组件,其中包含 `updateCurrentPage` 函数来更新当前页码:
```html
<template>
<div class="pagination">
<button @click="previousPage" :disabled="currentPage === 1">上一页</button>
<span>{{ currentPage }}</span>
<button @click="nextPage" :disabled="currentPage === totalPages">下一页</button>
</div>
</template>
<script>
export default {
props: {
totalItems: {
type: Number,
required: true
},
itemsPerPage: {
type: Number,
default: 10
}
},
computed: {
totalPages() {
return Math.ceil(this.totalItems / this.itemsPerPage);
}
},
data() {
return {
currentPage: 1
};
},
methods: {
previousPage() {
if (this.currentPage > 1) {
this.currentPage--;
this.updateCurrentPage();
}
},
nextPage() {
if (this.currentPage < this.totalPages) {
this.currentPage++;
this.updateCurrentPage();
}
},
updateCurrentPage() {
// 在这里执行更新当前页码的操作
console.log("当前页码是:" + this.currentPage);
}
}
};
</script>
```
该组件接收 `totalItems` 和 `itemsPerPage` 两个参数,分别表示总共的条目数和每页显示的条目数。在 `computed` 中计算出总页数 `totalPages`,在 `data` 中定义当前页码 `currentPage`,在 `methods` 中实现了上一页和下一页的点击事件,并在其中调用了 `updateCurrentPage` 函数来更新当前页码。
您可以在 `updateCurrentPage` 函数中添加其他操作来更新当前页码所对应的数据。在父组件中使用该分页组件时,需要传入 `totalItems` 和 `itemsPerPage` 两个参数,并监听 `updateCurrentPage` 事件来获取当前页码。例如:
```html
<template>
<div>
<my-pagination :total-items="totalItems" :items-per-page="itemsPerPage" @update-current-page="onUpdateCurrentPage" />
<!-- 在这里渲染当前页码所对应的数据 -->
</div>
</template>
<script>
import MyPagination from "./MyPagination.vue";
export default {
components: {
MyPagination
},
data() {
return {
totalItems: 100,
itemsPerPage: 10,
currentPage: 1
};
},
methods: {
onUpdateCurrentPage(page) {
this.currentPage = page;
// 在这里更新当前页码所对应的数据
}
}
};
</script>
```
在这个例子中,父组件监听了 `updateCurrentPage` 事件,当事件触发时将当前页码 `currentPage` 更新为事件传递过来的页码 `page`,并在 `onUpdateCurrentPage` 函数中更新当前页码所对应的数据。
this.partService .query({ page: pageToLoad - 1, size: this.itemsPerPage, sort: this.sort(), })以上代码中添加一个aaazi字段等于null 的条件怎么加
可以使用`HttpParams`的`append`方法来添加查询参数,其中包括`aaazi`字段等于`null`的条件。代码示例如下:
```typescript
import { HttpParams } from '@angular/common/http';
import { PartService } from './part.service';
// ...
constructor(private partService: PartService) {}
// ...
const pageToLoad = 1; // 页码
const params = new HttpParams()
.set('aaazi', 'null') // 添加 aaazi 字段等于 null 的条件
.set('page', (pageToLoad - 1).toString()) // 添加其他查询参数
.set('size', this.itemsPerPage.toString())
.set('sort', this.sort());
this.partService.query({ params }).subscribe(response => {
// 处理响应
});
```
在上面的代码中,使用`HttpParams`的`set`方法添加了名为`aaazi`,值为`null`的查询参数条件,同时添加了其他查询参数。在发送查询请求时,将该参数传递给`this.partService.query`方法的参数中。在后端处理请求时,可以根据该条件来筛选符合条件的数据。
相关推荐
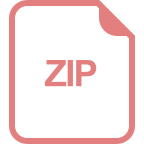
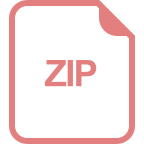
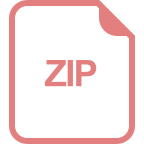







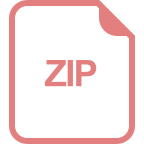
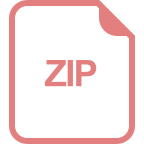
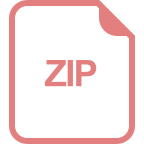
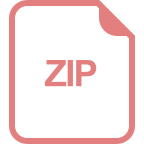
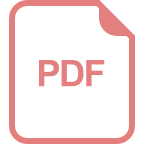
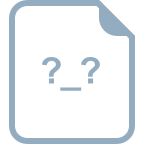