matlab农夫过河代码
时间: 2024-12-27 10:20:42 浏览: 10
### Matlab 实现农夫过河问题
#### 使用宽度优先搜索(BFS)
在解决经典的“农夫过河”问题时,可以采用宽度优先搜索(BFS)来遍历所有可能的状态组合直到找到解决方案。此方法能够有效地探索状态空间并记录路径。
下面是一个基于 BFS 的 MATLAB 代码示例:
```matlab
function farmer_cross_river()
% 初始化起始状态:所有人/物都在左岸
startState = [1, 1, 1, 1]; %[农民 左右;狼 左右;羊 左右;菜 左右]
% 定义目标状态:所有人都到了右岸
goalState = [0, 0, 0, 0];
% 可能的动作列表
actions = {[1, 1, 0, 0], ...% 带着狼过河
[1, 0, 1, 0], ...% 带着羊过河
[1, 0, 0, 1], ...% 带着菜过河
[1, 0, 0, 0]}; % 单独过河
queue = cell(1); % 创建队列用于存储待处理节点
visitedStates = {}; % 记录已访问过的状态防止重复计算
parentMap = containers.Map;% 存储父节点映射以便回溯解路径
enqueue(queue, {startState});
while ~isempty(queue)
currentState = dequeue(queue);
if isequal(currentState{1}, goalState)
break;
end
for actionIdx = 1:length(actions)
newState = update_state(currentState{1}, actions(actionIdx));
if isValid(newState) && ~ismember(newState, visitedStates,'rows')
enqueue(queue, {newState});
append(visitedStates, newState);
keyStr = mat2str(newState);
parentMap(keyStr) = mat2str(currentState{1});
end
end
end
reconstruct_path(parentMap, startState, goalState);
end
function result = update_state(state, move)
result = state - move .* (state == 1) + move .* (state ~= 1);
end
function valid = isValid(state)
% 判断当前状态是否合法
wolfSheepConflict = xor(state(2), state(3)); % 狼和绵羊冲突检测
sheepVegetableConflict = xor(state(3), state(4));% 绵羊和蔬菜冲突检测
aloneOnOppositeBank = any(state == all([0; 1])); % 是否有人单独留在对岸
valid = ~(wolfSheepConflict | sheepVegetableConflict | aloneOnOppositeBank);
end
function path = reconstruct_path(map, initialState, finalState)
currentKey = mat2str(finalState);
path = {};
while strcmp(currentKey, mat2str(initialState)) == false
prevKey = map(currentKey);
path{end+1} = str2num(prevKey);
currentKey = prevKey;
end
disp('Solution Path:');
for i=length(path):-1:1
fprintf('%d -> ',path{i})
end
fprintf('\n');
end
function enqueue(QueueCellArray, Element)
QueueCellArray{end+1}=Element;
end
function element = dequeue(QueueCellArray)
element = QueueCellArray{1};
QueueCellArray(1)=[]; %#ok<DEL cellular>
end
```
这段代码定义了一个 `farmer_cross_river` 函数,它通过广度优先搜索尝试解决问题,并打印出从初始位置到最终目的地的一系列动作序列[^1]。
阅读全文
相关推荐






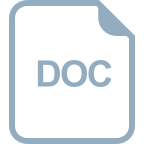







