4.Given an array (less than 100 in length) that may have duplicate values, find the minimum k non re
时间: 2024-11-10 15:14:08 浏览: 21
给定一个长度小于100的数组,其中可能存在重复值,目标是找到最小的k个非重复元素。这个问题通常被称为“找出数组中的前k个唯一元素”(Top K Frequent Elements)。你可以通过几种算法来解决:
1. **哈希表**:遍历数组,使用哈希表记录每个元素及其出现次数。然后,维护一个优先队列(堆),每次都将频率最低的元素添加到队列,并更新堆顶元素的计数。当堆大小达到k时,队列中的元素即为结果。
2. **排序+双指针**:首先对数组进行排序,然后使用两个指针,一个指向开始,另一个指向结束。比较当前指针所指元素的频率,如果它小于k,则移动频率先的指针;否则,移动结束指针。重复此过程直到两指针相遇。
3. **使用集合数据结构**:例如在Python中,可以利用collections模块的Counter类统计元素频率,再结合heapq库的heappushpop操作找到最小k个唯一元素。
```python
from collections import Counter
import heapq
def topKFrequent(nums, k):
counter = Counter(nums)
return heapq.nlargest(k, counter.keys(), key=counter.get)
```
相关问题
write a python code:City staff need to conduct statistics on the population information of the city, including name, gender, age, and community. There are duplicate values, missing values, and unreasonable values in the data. Please handle them as required: Duplicate values: name: if there are two names, keep the first one. Missing values: name: drop the line. gender: fill in Unknown. age: fill in 0. community: fill in Unknown. Unreasonable values: age: if the age is less than 0, fill in 0. Please output average age of each gender in each community.
Here is the Python code to handle the given requirements:
```python
import pandas as pd
# read the input data file
df = pd.read_csv('population_info.csv')
# remove duplicates based on name
df.drop_duplicates(subset=['name'], keep='first', inplace=True)
# drop rows with missing name values
df.dropna(subset=['name'], inplace=True)
# fill missing gender and community values with Unknown
df.fillna(value={'gender': 'Unknown', 'community': 'Unknown'}, inplace=True)
# fill negative age values with 0
df.loc[df['age'] < 0, 'age'] = 0
# calculate average age of each gender in each community
avg_age = df.groupby(['community', 'gender'])['age'].mean()
# print the result
print(avg_age)
```
Note: This code assumes that the input data file is in CSV format and is named "population_info.csv". You need to replace this with the actual file name.
There are duplicate values, missing values, and unreasonable values in the data. Please handle them as required: Duplicate values: name: if there are two names, keep the first one. Missing values:name: drop the line. gender: fill in Unknown. age: fill in 0. community: fill in Unknown. Unreasonable values:age: if the age is less than 0, fill in 0. Please output average age of each gender in each community. Input The input is the path name of the file. 1filename = input() Output print the output dataframe as sample. Samples sample.csv 1name,gender,age,community 2name2,F,31,F 3name5,M,12,F 4name6,F,88,E 5name3,M,18,G 6name9,F,85,D 7name5,M,87,G 8,M,26,C 9name2,F,60,F 10name2,F,89,F 11name0,F,15,E 12 Input sample.csv Output avg_age community gender D F 85.0 E F 51.5 F F 31.0 M 12.0 G M 18.0 use pandas in python
Sure, I understand your question. Here's the Python code to handle the given data as required using pandas:
```
import pandas as pd
# read the input file
filename = input("Enter the path of the file: ")
df = pd.read_csv(filename)
# handling duplicate values: keep the first one
df.drop_duplicates(subset=['name'], keep='first', inplace=True)
# handling missing values: drop the line
df.dropna(subset=['name'], inplace=True)
df['gender'].fillna(value='Unknown', inplace=True)
df['age'].fillna(value=0, inplace=True)
df['community'].fillna(value='Unknown', inplace=True)
# handling unreasonable values: fill age less than 0 with 0
df.loc[df['age'] < 0, 'age'] = 0
# group by community and gender, calculate average age
df_grouped = df.groupby(['community', 'gender']).mean()
df_output = df_grouped[['age']] # select only the 'age' column
# print the output dataframe
print(df_output)
```
This code should handle the given data as required, and output the average age of each gender in each community.
阅读全文
相关推荐
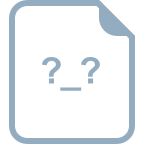
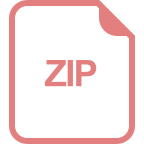








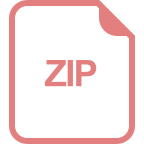