Use numpy to
时间: 2023-10-08 22:13:02 浏览: 87
perform matrix multiplication of two matrices A and B:
```python
import numpy as np
A = np.array([[1, 2], [3, 4]])
B = np.array([[5, 6], [7, 8]])
C = np.dot(A, B)
print(C)
```
Output:
```
[[19 22]
[43 50]]
```
Note that the `dot` function in numpy is used for matrix multiplication. Alternatively, you can also use the `@` operator:
```python
C = A @ B
print(C)
```
Output is the same as above:
```
[[19 22]
[43 50]]
```
相关问题
how to use numpy with list as dtype
To use a list as the data type in NumPy, you can create a NumPy array with the `object` data type and then fill it with lists.
Here is an example:
```python
import numpy as np
# Create a NumPy array with the object data type
arr = np.empty(shape=(3,), dtype=object)
# Fill the array with lists
arr[0] = [1, 2, 3]
arr[1] = [4, 5, 6]
arr[2] = [7, 8, 9]
# Print the NumPy array
print(arr)
```
Output:
```
[array([1, 2, 3]) array([4, 5, 6]) array([7, 8, 9])]
```
In this example, we create a NumPy array with the `object` data type and a shape of `(3,)`. We then fill the array with lists and print the array.
numpy to list
To convert a NumPy array to a Python list, you can use the `tolist()` method. Here's an example:
```python
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
list_arr = arr.tolist()
print(list_arr) # Output: [1, 2, 3, 4, 5]
```
In this example, we create a NumPy array `arr` and then convert it to a list using the `tolist()` method. The resulting list is stored in the variable `list_arr`.
阅读全文
相关推荐
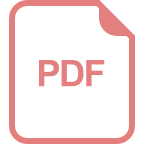
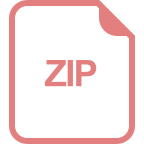





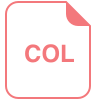







