springboot导入excel数据
时间: 2023-06-05 10:48:05 浏览: 150
Spring Boot可以通过Apache POI库来导入Excel数据。具体步骤如下:
1. 在pom.xml文件中添加Apache POI依赖:
```xml
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
```
2. 创建一个Excel文件读取工具类,使用POI库读取Excel文件数据并返回一个List对象:
```java
import org.apache.poi.ss.usermodel.*;
import java.io.File;
import java.io.FileInputStream;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class ExcelReader {
public static List<List<String>> readExcel(File file) throws Exception {
List<List<String>> result = new ArrayList<>();
// 创建工作簿
Workbook workbook = WorkbookFactory.create(new FileInputStream(file));
// 获取第一个工作表
Sheet sheet = workbook.getSheetAt();
// 遍历行
Iterator<Row> rowIterator = sheet.rowIterator();
while (rowIterator.hasNext()) {
Row row = rowIterator.next();
// 遍历单元格
Iterator<Cell> cellIterator = row.cellIterator();
List<String> rowList = new ArrayList<>();
while (cellIterator.hasNext()) {
Cell cell = cellIterator.next();
rowList.add(cell.toString());
}
result.add(rowList);
}
return result;
}
}
```
3. 在Controller中调用ExcelReader类的readExcel方法,将Excel文件转换为List对象:
```java
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import java.io.File;
import java.util.List;
@RestController
public class ExcelController {
@PostMapping("/upload")
public List<List<String>> upload(@RequestParam("file") MultipartFile file) throws Exception {
File tempFile = File.createTempFile("temp", ".xlsx");
file.transferTo(tempFile);
return ExcelReader.readExcel(tempFile);
}
}
```
以上就是使用Spring Boot导入Excel数据的步骤。
相关推荐
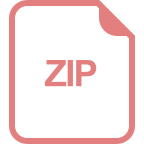














