请用C++写一个顺序查找和折半查找
时间: 2024-02-09 15:10:34 浏览: 73
顺序查找(线性查找):
```c++
#include <iostream>
using namespace std;
int seqSearch(int arr[], int n, int x) {
for (int i = 0; i < n; i++) {
if (arr[i] == x) {
return i;
}
}
return -1;
}
int main() {
int arr[] = {2, 3, 5, 7, 11, 13, 17, 19};
int n = sizeof(arr) / sizeof(arr[0]);
int x = 11;
int index = seqSearch(arr, n, x);
if (index != -1) {
cout << "Found at index " << index << endl;
} else {
cout << "Not found" << endl;
}
return 0;
}
```
折半查找(二分查找):
```c++
#include <iostream>
using namespace std;
int binarySearch(int arr[], int n, int x) {
int left = 0, right = n - 1;
while (left <= right) {
int mid = (left + right) / 2;
if (arr[mid] == x) {
return mid;
} else if (arr[mid] > x) {
right = mid - 1;
} else {
left = mid + 1;
}
}
return -1;
}
int main() {
int arr[] = {2, 3, 5, 7, 11, 13, 17, 19};
int n = sizeof(arr) / sizeof(arr[0]);
int x = 11;
int index = binarySearch(arr, n, x);
if (index != -1) {
cout << "Found at index " << index << endl;
} else {
cout << "Not found" << endl;
}
return 0;
}
```
阅读全文
相关推荐
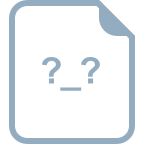
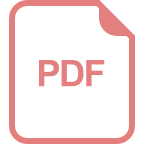















