<?xml version="1.0" encoding="utf-8"?> <TableLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/tableLayout1" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="@drawable/background"> <TableRow android:id="@+id/tableRow1" android:layout_width="wrap_content" android:layout_height="wrap_content"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:inputType="textEmailAddress" android:text="会员昵称:" android:height="50px" /> <EditText android:id="@+id/nickname" android:hint="请输入会员昵称" android:layout_width="300px" android:layout_height="wrap_content" android:singleLine="true" /> </TableRow> <TableRow android:id="@+id/tableRow2" android:layout_width="wrap_content" android:layout_height="wrap_content"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:inputType="textEmailAddress" android:text="输入密码:" android:height="50px" /> <EditText android:id="@+id/pwd" android:layout_width="300px" android:inputType="textPassword" android:layout_height="wrap_content" /> </TableRow> <TableRow android:id="@+id/tableRow3" android:layout_width="wrap_content" android:layout_height="wrap_content"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:inputType="textEmailAddress" android:text="确认密码:" android:height="50px" /> <EditText android:id="@+id/repwd" android:layout_width="300px" android:layout_height="wrap_content" android:inputType="textPassword" /> </TableRow> <TableRow android:id="@+id/tableRow4" android:layout_width="wrap_content" android:layout_height="wrap_content"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:inputType="textEmailAddress" android:text="E-mail:" android:height="50px" /> <EditText android:id="@+id/email" android:layout_width="300px" android:layout_height="wrap_content" android:inputType="textEmailAddress" /> </TableRow> <LinearLayout android:orientation="horizontal" android:layout_width="wrap_content" android:layout_height="wrap_content" > <Button android:text="注册" android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content"/> <Button android:text="重置" android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content"/> </LinearLayout> </TableLayout>详细解释下这段代码的作用
时间: 2023-07-19 09:46:56 浏览: 1621
这段代码是一个 Android 应用程序中的布局文件,使用 TableLayout 和 TableRow 来创建一个表格。其中,每个 TableRow 包含一个 TextView 和一个 EditText,用于输入用户的昵称、密码和电子邮件地址。LinearLayout 中包含两个 Button,用于触发注册和重置操作。此外,还指定了布局的背景图。
相关问题
android TableLayout 单双背景
你可以通过在 TableLayout 中的每个 TableRow 中设置不同的背景来实现单双行不同的背景效果。具体步骤如下:
1. 在 res/drawable 目录下创建两个 XML 文件,分别命名为 odd_bg.xml 和 even_bg.xml。这两个文件分别代表了奇数行和偶数行的背景。
2. 在 odd_bg.xml 中添加以下内容:
```xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<solid android:color="@color/odd_color" />
</shape>
```
其中,@color/odd_color 代表了奇数行的背景颜色。
3. 在 even_bg.xml 中添加以下内容:
```xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<solid android:color="@color/even_color" />
</shape>
```
其中,@color/even_color 代表了偶数行的背景颜色。
4. 在 TableLayout 中添加每个 TableRow 的时候,判断当前行是奇数行还是偶数行,然后设置不同的背景。
```java
for (int i = 0; i < rowCount; i++) {
TableRow tableRow = new TableRow(this);
// 判断当前行是奇数行还是偶数行
if (i % 2 == 0) {
tableRow.setBackgroundResource(R.drawable.even_bg);
} else {
tableRow.setBackgroundResource(R.drawable.odd_bg);
}
// 添加其他控件到 TableRow 中
...
tableLayout.addView(tableRow);
}
```
其中,R.drawable.even_bg 和 R.drawable.odd_bg 分别对应了上面创建的两个 XML 文件。
这样就可以实现单双行不同的背景效果了。
android tableLayout使用实例
好的,下面是一个简单的 Android TableLayout 的使用示例:
1. 创建一个 layout 文件:
```xml
<?xml version="1.0" encoding="utf-8"?>
<TableLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<!-- 第一行 -->
<TableRow>
<TextView
android:text="姓名"
android:textStyle="bold" />
<TextView
android:text="年龄"
android:textStyle="bold" />
<TextView
android:text="性别"
android:textStyle="bold" />
</TableRow>
<!-- 第二行 -->
<TableRow>
<TextView
android:text="张三" />
<TextView
android:text="18" />
<TextView
android:text="男" />
</TableRow>
<!-- 第三行 -->
<TableRow>
<TextView
android:text="李四" />
<TextView
android:text="20" />
<TextView
android:text="女" />
</TableRow>
</TableLayout>
```
2. 在 Activity 中设置 ContentView:
```java
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
}
```
就可以在界面上显示一个简单的表格了。其中,TableLayout 是表格的总容器,TableRow 是每一行的容器,TextView 是表格中的单元格。在 TableRow 中添加 TextView,即可创建表格的内容。
阅读全文
相关推荐
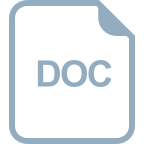
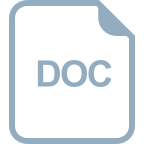
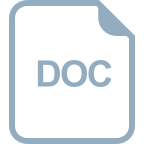
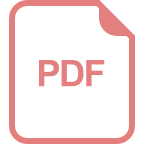
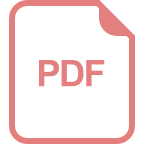
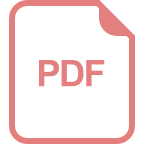
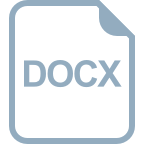
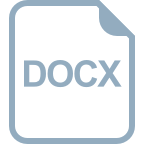
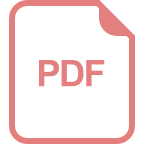
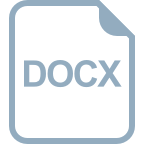
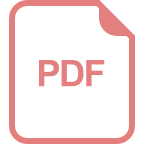
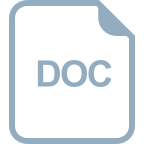
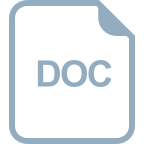
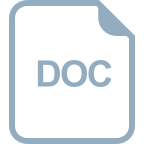
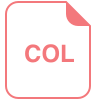
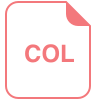
