class MyBertModel(nn.Module): def __init__(self, config): super(MyBertModel, self).__init__() self.bert = BertModel.from_pretrained(config.bert_path) for param in self.bert.parameters(): param.requires_grad = True self.fc = nn.Linear(config.hidden_size, config.num_classes) def forward(self, x): context = x[0] # 输入的句子 mask = x[2] # 对padding部分进行mask,和句子一个size,padding部分用0表示,如:[1, 1, 1, 1, 0, 0] _, pooled = self.bert(context, attention_mask=mask, output_all_encoded_layers=False) out = self.fc(pooled) return out
时间: 2024-04-28 10:25:32 浏览: 10
这是一个 PyTorch 的模型类,名为 `MyBertModel`,继承自 `nn.Module`。这个模型使用了预训练的 BERT 模型,通过 `BertModel.from_pretrained(config.bert_path)` 加载预训练模型。然后,使用一个全连接层 `nn.Linear` 将 BERT 的输出映射到指定的类别个数上,即 `config.num_classes`。
在 forward 方法中,输入 `x` 是一个元组,包含三个张量:句子的 token id 序列 `context`,对应的 token 序列长度 `seq_len`,以及 padding 部分的掩码 `mask`。使用 BERT 模型对 `context` 序列进行编码,得到输出 `pooled`。最后,将 `pooled` 传入全连接层 `self.fc` 得到输出结果 `out`,并返回。
相关问题
class Pointnet2MSG(nn.Module): def __init__(self, input_channels=6, use_xyz=True): super().__init__() self.SA_modules = nn.ModuleList() channel_in = input_channels skip_channel_list = [input_channels] for k in range(cfg.RPN.SA_CONFIG.NPOINTS.__len__()): mlps = cfg.RPN.SA_CONFIG.MLPS[k].copy() channel_out = 0 for idx in range(mlps.__len__()): mlps[idx] = [channel_in] + mlps[idx] channel_out += mlps[idx][-1] self.SA_modules.append( PointnetSAModuleMSG( npoint=cfg.RPN.SA_CONFIG.NPOINTS[k], radii=cfg.RPN.SA_CONFIG.RADIUS[k], nsamples=cfg.RPN.SA_CONFIG.NSAMPLE[k], mlps=mlps, use_xyz=use_xyz, bn=cfg.RPN.USE_BN ) ) skip_channel_list.append(channel_out) channel_in = channel_out这是我改进之前的类代码块,而这是我加入SA注意力机制后的代码块:class Pointnet2MSG(nn.Module): def __init__(self, input_channels=6, use_xyz=True): super().__init__() self.SA_modules = nn.ModuleList() channel_in = input_channels skip_channel_list = [input_channels] for k in range(cfg.RPN.SA_CONFIG.NPOINTS.__len__()): mlps = cfg.RPN.SA_CONFIG.MLPS[k].copy() channel_out = 0 for idx in range(mlps.__len__()): mlps[idx] = [channel_in] + mlps[idx] channel_out += mlps[idx][-1] mlps.append(channel_out) self.SA_modules.append( nn.Sequential( PointnetSAModuleMSG( npoint=cfg.RPN.SA_CONFIG.NPOINTS[k], radii=cfg.RPN.SA_CONFIG.RADIUS[k], nsamples=cfg.RPN.SA_CONFIG.NSAMPLE[k], mlps=mlps, use_xyz=use_xyz, bn=cfg.RPN.USE_BN, ), SelfAttention(channel_out) ) ) skip_channel_list.append(channel_out) channel_in = channel_out,我发现改进后的代码块对于mlps参数的计算非常混乱,请你帮我检查一下,予以更正并给出注释
在你加入SA注意力机制后的代码块中,你在计算mlps时,添加了一个长度为1的列表,这个列表的元素为channel_out,这样做的目的是为了在SA模块中使用channel_out作为输入特征的通道数。但是这样的话,你的mlps列表就不再是每一层的输出通道数了,而是包含了一个额外的元素channel_out。
为了解决这个问题,你可以在SA模块中获取mlps列表中除了最后一个元素以外的所有元素,并将它们作为SA模块的输入通道数,最后将SA模块的输出通道数设置为mlps列表中最后一个元素即可。
以下是修改后的代码块,已经添加了注释:
```
class Pointnet2MSG(nn.Module):
def __init__(self, input_channels=6, use_xyz=True):
super().__init__()
self.SA_modules = nn.ModuleList()
channel_in = input_channels
skip_channel_list = [input_channels]
for k in range(cfg.RPN.SA_CONFIG.NPOINTS.__len__()):
mlps = cfg.RPN.SA_CONFIG.MLPS[k].copy()
channel_out = 0
for idx in range(mlps.__len__()):
mlps[idx] = [channel_in] + mlps[idx]
channel_out += mlps[idx][-1]
# 移除mlps列表中的最后一个元素,并将其作为SA模块的输出通道数
sa_channel_out = mlps.pop()
self.SA_modules.append(
nn.Sequential(
PointnetSAModuleMSG(
npoint=cfg.RPN.SA_CONFIG.NPOINTS[k],
radii=cfg.RPN.SA_CONFIG.RADIUS[k],
nsamples=cfg.RPN.SA_CONFIG.NSAMPLE[k],
mlps=mlps,
use_xyz=use_xyz,
bn=cfg.RPN.USE_BN,
),
SelfAttention(sa_channel_out, sa_channel_out // 8) # 通常将隐藏层大小设置为输出通道数的1/8
)
)
skip_channel_list.append(sa_channel_out)
channel_in = sa_channel_out
```
class EntityRankerClassifier(nn.Module): def __init__(self, n_classes, PRE_TRAINED_MODEL_NAME): super(EntityRankerClassifier, self).__init__() self.bert = AutoModel.from_pretrained(PRE_TRAINED_MODEL_NAME) self.drop = nn.Dropout(p=0.3) self.out = nn.Linear(self.bert.config.hidden_size, n_classes) def forward(self, input_ids, attention_mask): _, pooled_output = self.bert( input_ids=input_ids, attention_mask=attention_mask, return_dict=False ) output = self.drop(pooled_output) return self.out(output)
这是一个使用预训练的BERT模型进行分类的PyTorch模型,具体来说,该模型包含以下几个部分:
1. 初始化函数:接受两个参数,一个是输出类别数n_classes,一个是预训练模型的名称PRE_TRAINED_MODEL_NAME,通过调用父类初始化函数来初始化模型。
2. 模型结构:该模型使用预训练的BERT模型作为编码器,通过AutoModel.from_pretrained函数加载预训练模型,并将输入的文本序列input_ids和注意力掩码attention_mask传入BERT模型中,得到BERT模型的输出。这里使用的是BERT模型的池化输出pooled_output,即将所有单词的输出取平均得到的一维向量,作为文本的表示。接着通过一个Dropout层进行正则化,最后通过一个全连接层进行分类。
3. 前向传播函数:接受两个参数,一个是文本序列input_ids,一个是注意力掩码attention_mask,将这两个参数传入模型结构中,得到模型的输出。
需要注意的是,在使用该模型进行分类时,需要将输入的文本序列转换为对应的input_ids和attention_mask,可以使用tokenizer将文本序列转换为模型所需的格式。在训练模型时,需要使用交叉熵损失函数和优化器对模型进行训练。
相关推荐
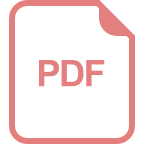











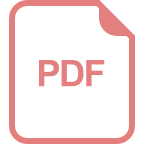
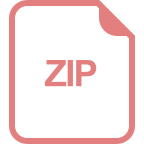