.实现基于有序顺序表的折半查找算法。 代码
时间: 2023-12-10 21:41:54 浏览: 104
以下是基于有序顺序表的折半查找算法的代码实现:
```c++
#include <iostream>
using namespace std;
int binarySearch(int arr[], int left, int right, int target) {
while (left <= right) {
int mid = (left + right) / 2;
if (arr[mid] == target) {
return mid;
}
else if (arr[mid] < target) {
left = mid + 1;
}
else {
right = mid - 1;
}
}
return -1;
}
int main() {
int arr[] = { 1, 3, 5, 7, 9, 11, 13, 15, 17, 19 };
int n = sizeof(arr) / sizeof(arr[0]);
int target = 11;
int result = binarySearch(arr, 0, n - 1, target);
if (result == -1) {
cout << "Element not found" << endl;
}
else {
cout << "Element found at index " << result << endl;
}
return 0;
}
```
在以上代码中,我们首先定义了一个 `binarySearch` 函数,它接受一个有序数组、左边界、右边界和目标元素作为参数,并返回目标元素在数组中的索引,如果目标元素不存在数组中,则返回 -1。
在 `binarySearch` 函数中,我们使用了一个 while 循环来不断缩小搜索范围,直到找到目标元素或者发现它不存在于数组中。我们首先计算中间元素的索引,然后与目标元素进行比较。如果中间元素等于目标元素,则我们已经找到了它,返回它的索引。如果中间元素小于目标元素,则目标元素可能存在于右侧,我们更新左边界为中间元素的下一个位置。如果中间元素大于目标元素,则目标元素可能存在于左侧,我们更新右边界为中间元素的上一个位置。这样,每次迭代都会将搜索范围减半,直到找到目标元素或者搜索范围为空。
在主函数中,我们定义了一个有序数组 `arr`,并计算出它的长度。然后,我们指定要搜索的目标元素 `target`,并将它作为参数传递给 `binarySearch` 函数。如果该函数返回 -1,则说明目标元素不存在于数组中,否则它将返回目标元素的索引。最后,我们根据函数的返回值输出相应的消息。
阅读全文
相关推荐




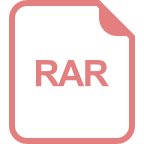
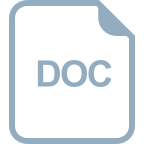
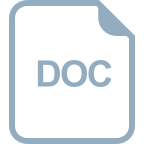
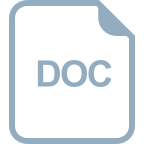
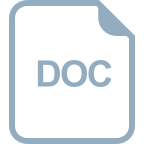
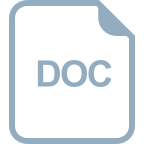





