"python中todense()函数是什么意思?"
时间: 2024-04-22 16:26:32 浏览: 25
`todense()`函数是NumPy库中的一个函数,它用于将稀疏矩阵转换为密集矩阵。在机器学习和数据分析,我们经常使用稀疏矩阵来表示大规模数据集,其中很多元素都是零。稀疏矩阵只存储非零元素的值和位置,以节省存储空间。但有时候我们需要将稀疏矩阵转换为密集矩阵,以便进行一些操作或计算,这时就可以使用`todense()`函数。它会将稀疏矩阵转换为一个完整的NumPy数组,其中包含了原始矩阵的所有元素。
相关问题
monai 是python的什么包
MONAI(Medical Open Network for AI)是一个基于Python的开源软件包,用于医学图像分析的深度学习任务。它提供了一系列工具和算法,用于处理和分析医学图像数据,帮助研究人员和开发者在医学影像领域进行深度学习模型的开发和部署。
MONAI支持各种医学图像任务,包括图像分割、分类、生成等。它提供了丰富的预处理和后处理函数,用于数据增强、标准化、裁剪等操作,以提高深度学习模型的性能和鲁棒性。此外,MONAI还提供了一系列用于构建、训练和评估模型的工具和API,使得医学图像分析变得更加简单和高效。
您可以使用pip命令来安装MONAI包:
```shell
pip install monai
```
一旦安装完成,您就可以在Python代码中导入MONAI并开始使用它来处理和分析医学图像数据了。例如:
```python
import monai
# 使用MONAI进行医学图像数据的加载和预处理
data = monai.utils.nib.load_image("image.nii.gz")
data = monai.transforms.RescaleIntensity()(data)
data = monai.transforms.ToTensor()(data)
# 构建和训练深度学习模型
model = monai.networks.nets.DenseNet(spatial_dims=3, in_channels=1, out_channels=2)
trainer = monai.engines.SupervisedTrainer(model, optimizer, loss_function)
trainer.fit(data_loader)
```
通过MONAI,您可以更轻松地处理和分析医学图像数据,加速医学影像领域的深度学习研究与应用。
用python写一个densenet网络模型
### 回答1:
我可以提供一个简单的Python示例,用于构建DenseNet网络模型:# 导入必要的库
import keras
from keras.layers import Dense, Conv2D, BatchNormalization, Activation
from keras.layers import AveragePooling2D, Input, Flatten
from keras.regularizers import l2
from keras.models import Model# 定义Dense Block
def dense_block(x, blocks, name):
"""A dense block.
# Arguments
x: input tensor.
blocks: integer, the number of building blocks.
name: string, block label.
# Returns
output tensor for the block.
"""
for i in range(blocks):
x = conv_block(x, 32, name=name + '_block' + str(i + 1))
return x# 定义构建densenet网络模型
def DenseNet(input_shape, classes):
"""Instantiates the DenseNet architecture.
# Arguments
input_shape: optional shape tuple, only to be specified
if `include_top` is False (otherwise the input shape
has to be `(32, 32, 3)` (with `channels_last` data format)
or `(3, 32, 32)` (with `channels_first` data format).
It should have exactly 3 inputs channels,
and width and height should be no smaller than 8.
E.g. `(200, 200, 3)` would be one valid value.
classes: The number of classes to classify images
into, only to be specified if `include_top` is True, and
if no `weights` argument is specified.
# Returns
A Keras model instance.
"""
# 定义输入
inputs = Input(shape=input_shape) # 调用dense block
x = dense_block(inputs, 4, name='dense_1') # 全局平均池化层
x = AveragePooling2D(pool_size=7, strides=None,
padding='same')(x) # 展平层
x = Flatten()(x) # 全连接层
outputs = Dense(classes,
activation='softmax',
kernel_initializer='he_normal')(x) # 创建模型
model = Model(inputs, outputs)
return model
### 回答2:
DenseNet是一种深度学习网络模型,其主要特点是具有密集连接的结构,通过在每个层级中将输入连接到后续层级中的所有前馈路径,旨在解决深层网络梯度消失和特征重复利用的问题。下面是用Python编写DenseNet网络模型的基本步骤:
1. 导入所需的Python库,例如NumPy、Keras和TensorFlow等。
2. 定义DenseNet网络模型的主体结构。DenseNet由多个密集块(dense block)组成。每个密集块中包含若干个相互连接的卷积层。在每个密集块的后面是一个过渡层(transition layer),用于减小输出特征图的维度。
3. 在每个密集块内部,定义相互连接的卷积层。这些卷积层通常由一个Batch Normalization层、一个ReLU激活函数和一个卷积层组成。
4. 在相邻的层之间进行连接,将前一个层的输出作为后一个层的输入,以实现密集连接的结构。
5. 在所需的输出层之前,最后添加全局平均池化层用于降低特征图的维度。
6. 编译和训练模型。设置损失函数和优化器,并使用适当的训练数据进行训练。
7. 评估模型的性能。使用测试数据对模型进行评估,计算准确率、损失等指标。
8. 使用模型进行预测。输入新的数据样本,使用已经训练好的模型进行预测。
需要注意的是,以上仅为DenseNet网络模型的基本步骤,具体的实现细节可能因使用的深度学习库和数据集而有所不同。编写DenseNet模型时,还需根据具体需求和数据集调整网络结构和超参数,以获得更好的性能。
### 回答3:
import torch
import torch.nn as nn
class BottleneckLayer(nn.Module):
def __init__(self, in_channels, growth_rate):
super(BottleneckLayer, self).__init__()
self.bn1 = nn.BatchNorm2d(in_channels)
self.relu1 = nn.ReLU(inplace=True)
self.conv1 = nn.Conv2d(in_channels, 4 * growth_rate, kernel_size=1, bias=False)
self.bn2 = nn.BatchNorm2d(4 * growth_rate)
self.relu2 = nn.ReLU(inplace=True)
self.conv2 = nn.Conv2d(4 * growth_rate, growth_rate, kernel_size=3, padding=1, bias=False)
def forward(self, x):
identity = x
out = self.bn1(x)
out = self.relu1(out)
out = self.conv1(out)
out = self.bn2(out)
out = self.relu2(out)
out = self.conv2(out)
out = torch.cat([identity, out], dim=1)
return out
class DenseBlock(nn.Module):
def __init__(self, in_channels, num_layers, growth_rate):
super(DenseBlock, self).__init__()
self.layers = nn.ModuleList([BottleneckLayer(in_channels + i * growth_rate, growth_rate) for i in range(num_layers)])
def forward(self, x):
for layer in self.layers:
x = layer(x)
return x
class TransitionLayer(nn.Module):
def __init__(self, in_channels, out_channels):
super(TransitionLayer, self).__init__()
self.bn = nn.BatchNorm2d(in_channels)
self.relu = nn.ReLU(inplace=True)
self.conv = nn.Conv2d(in_channels, out_channels, kernel_size=1, bias=False)
self.avg_pool = nn.AvgPool2d(kernel_size=2, stride=2)
def forward(self, x):
out = self.bn(x)
out = self.relu(out)
out = self.conv(out)
out = self.avg_pool(out)
return out
class DenseNet(nn.Module):
def __init__(self, block_config, growth_rate=32, num_classes=1000):
super(DenseNet, self).__init__()
self.conv1 = nn.Conv2d(3, 64, kernel_size=7, stride=2, padding=3, bias=False)
self.relu = nn.ReLU(inplace=True)
self.max_pool = nn.MaxPool2d(kernel_size=3, stride=2, padding=1)
self.dense_block_1 = self._make_dense_block(block_config[0], growth_rate)
self.transition_1 = self._make_transition_layer(64 + block_config[0] * growth_rate, growth_rate)
self.dense_block_2 = self._make_dense_block(block_config[1], growth_rate)
self.transition_2 = self._make_transition_layer(64 + block_config[0] * growth_rate + block_config[1] * growth_rate, growth_rate)
self.dense_block_3 = self._make_dense_block(block_config[2], growth_rate)
self.transition_3 = self._make_transition_layer(64 + block_config[0] * growth_rate + block_config[1] * growth_rate + block_config[2] * growth_rate, growth_rate)
self.dense_block_4 = self._make_dense_block(block_config[3], growth_rate)
self.avg_pool = nn.AdaptiveAvgPool2d((1, 1))
self.fc = nn.Linear(64 + block_config[0] * growth_rate + block_config[1] * growth_rate + block_config[2] * growth_rate + block_config[3] * growth_rate, num_classes)
def forward(self, x):
out = self.conv1(x)
out = self.relu(out)
out = self.max_pool(out)
out = self.dense_block_1(out)
out = self.transition_1(out)
out = self.dense_block_2(out)
out = self.transition_2(out)
out = self.dense_block_3(out)
out = self.transition_3(out)
out = self.dense_block_4(out)
out = self.avg_pool(out)
out = torch.flatten(out, 1)
out = self.fc(out)
return out
def _make_dense_block(self, num_layers, growth_rate):
layers = []
for i in range(num_layers):
layers.append(BottleneckLayer(growth_rate, growth_rate))
return DenseBlock(growth_rate, num_layers, growth_rate)
def _make_transition_layer(self, in_channels, out_channels):
return TransitionLayer(in_channels, out_channels)
# 模型参数设置
block_config = [6, 12, 24, 16]
growth_rate = 32
num_classes = 1000
# 创建DenseNet模型
model = DenseNet(block_config, growth_rate, num_classes)
# 打印模型结构
print(model)
使用Python编写了一个DenseNet网络模型。DenseNet是一种卷积神经网络架构,具有低延迟和高精度的特点。该模型由多个DenseBlock组成,其中每个DenseBlock由多个BottleneckLayer堆叠而成,以增加网络的深度和复杂性。每个BottleneckLayer由BatchNormalization、ReLU和Convolutional层构成。通过连接之前的特征图和当前层的输出,实现了密集连接,使得模型能够充分利用之前层的特征信息。在DenseBlock之间,使用TransitionLayer进行降维和下采样操作,以减小网络参数和内存的消耗。最后通过全局平均池化、全连接层和Softmax函数将输出映射到指定的类别上。
相关推荐
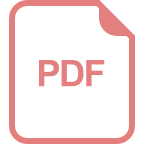
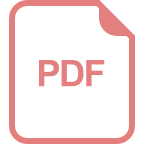












