c++多线程与函数指针
时间: 2023-11-22 11:43:22 浏览: 77
好的,您的问题是:
在 C 中如何使用多线程和函数指针?
答案:
在 C 中,使用多线程需要使用线程库,例如 pthread。使用 pthread 库可以通过创建线程来实现多线程编程。下面是一个使用 pthread 创建线程的例子:
```c
#include <stdio.h>
#include <pthread.h>
void *my_func(void *arg) {
printf("Hello from thread!\n");
return NULL;
}
int main() {
pthread_t tid;
pthread_create(&tid, NULL, my_func, NULL);
pthread_join(tid, NULL);
printf("Thread finished.\n");
return 0;
}
```
在上面的例子中,`pthread_create` 函数用于创建一个新的线程,`my_func` 函数是新线程将要执行的函数。`pthread_join` 函数用于等待新线程结束。
关于函数指针,在 C 中我们可以通过定义函数指针变量来调用函数。例如:
```c
#include <stdio.h>
void my_func(int arg) {
printf("my_func is called with arg=%d\n", arg);
}
int main() {
void (*func_ptr)(int);
func_ptr = my_func;
func_ptr(123);
return 0;
}
```
在上面的例子中,我们定义了一个函数指针变量 `func_ptr`,并将其赋值为 `my_func` 函数。然后,我们可以直接调用函数指针变量来调用 `my_func` 函数。
阅读全文
相关推荐
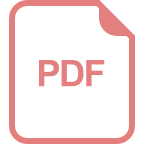
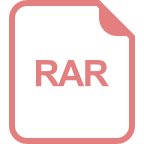
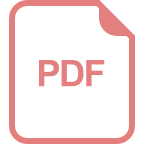
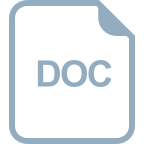
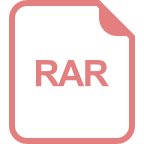
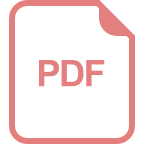
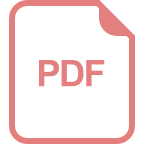
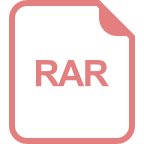
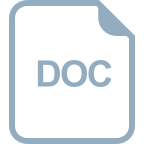
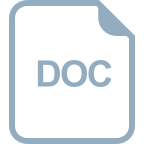
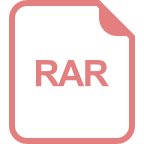
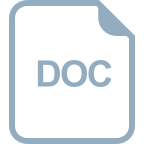
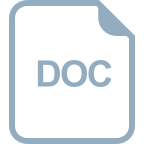
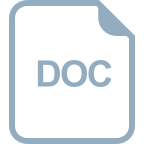
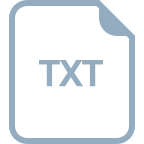