linux如何用c语言清空一个文件
时间: 2024-05-13 14:18:56 浏览: 19
在 Linux 中,可以使用 C 语言的标准库函数 `fopen()` 和 `fclose()` 打开和关闭文件,使用 `ftruncate()` 函数清空一个文件。
下面是一个示例程序,展示如何清空一个文件:
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
FILE *file = fopen("example.txt", "w");
if (file == NULL) {
perror("Failed to open file");
return 1;
}
if (ftruncate(fileno(file), 0) != 0) {
perror("Failed to truncate file");
fclose(file);
return 1;
}
fclose(file);
return 0;
}
```
在上面的示例程序中,首先使用 `fopen()` 函数打开一个文件,并指定以写入模式打开它。然后,使用 `fileno()` 函数获取文件描述符,将其作为第一个参数传递给 `ftruncate()` 函数,第二个参数传递为 0,表示将文件截断为 0 字节。最后,使用 `fclose()` 函数关闭文件。
相关问题
linux中C语言fopen怎么使用
在 Linux 系统中,使用 C 语言中的 `fopen` 函数打开文件的语法如下:
```c
#include <stdio.h>
FILE *fopen(const char *filename, const char *mode);
```
其中,`filename` 参数是要打开的文件名,可以是相对路径或绝对路径;`mode` 参数则是打开文件的模式,包括:
- `"r"`:只读模式,打开文件用于读取。
- `"w"`:只写模式,创建一个空文件用于写入。如果文件已经存在,则会清空文件内容。
- `"a"`:追加模式,打开一个文件用于写入。如果文件已经存在,则在文件末尾追加内容。
- `"r+"`:读写模式,打开一个文件用于读取和写入。
- `"w+"`:读写模式,创建一个空文件用于读取和写入。如果文件已经存在,则会清空文件内容。
- `"a+"`:读写模式,打开一个文件用于读取和追加内容。
函数会返回一个指向 `FILE` 类型的文件指针,如果文件打开失败,则返回 `NULL`。打开文件后,可以使用 `fread` 和 `fwrite` 函数进行读写操作,最后使用 `fclose` 函数关闭文件。
以下是一个示例代码:
```c
#include <stdio.h>
int main() {
FILE *fp;
char buf[1024];
fp = fopen("test.txt", "r"); // 打开文件
if (fp == NULL) {
printf("Failed to open file.\n");
return -1;
}
// 读取文件内容
while (fgets(buf, sizeof(buf), fp) != NULL) {
printf("%s", buf);
}
fclose(fp); // 关闭文件
return 0;
}
```
用c语言写一个会动的爱心
以下是一个简单的用C语言编写的会动的爱心动画,你可以在控制台中看到它:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
int main()
{
while (1) {
system("clear"); // 清空屏幕
for (int i = -10; i <= 10; i++) {
for (int j = -10; j <= 10; j++) {
if ((i * i + j * j) <= 100) {
printf("❤️ ");
} else {
printf(" ");
}
}
printf("\n");
}
usleep(300000); // 等待一段时间
}
return 0;
}
```
在Linux或MacOS上,你可以将代码保存为`heart.c`文件,然后在终端中运行以下命令来编译它:
```
gcc heart.c -o heart
```
最后,你可以通过运行以下命令来启动动画:
```
./heart
```
当你看到动画时,你可以按`Ctrl+C`来停止它。
相关推荐
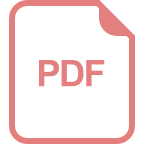
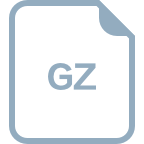
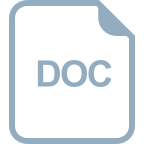
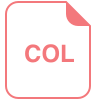











