使用c语言 编程模拟洗牌和发牌过程
时间: 2023-08-09 21:10:12 浏览: 93
以下是一个简单的洗牌和发牌程序的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define NUM_CARDS 52
#define NUM_RANKS 13
#define NUM_SUITS 4
enum suit { CLUBS, DIAMONDS, HEARTS, SPADES };
enum rank { ACE = 1, TWO, THREE, FOUR, FIVE, SIX, SEVEN, EIGHT, NINE, TEN, JACK, QUEEN, KING };
typedef struct {
int rank;
int suit;
} card;
void shuffle(card *deck) {
int i, j;
card temp;
for (i = NUM_CARDS - 1; i > 0; i--) {
j = rand() % (i + 1); // 随机选择一张牌
temp = deck[i];
deck[i] = deck[j];
deck[j] = temp;
}
}
void print_card(card c) {
const char *ranks[] = { "Ace", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine", "Ten", "Jack", "Queen", "King" };
const char *suits[] = { "Clubs", "Diamonds", "Hearts", "Spades" };
printf("%s of %s\n", ranks[c.rank - 1], suits[c.suit]);
}
int main() {
card deck[NUM_CARDS];
int i, num_players;
srand((unsigned) time(NULL)); // 使用当前时间作为随机种子
// 初始化牌堆
for (i = 0; i < NUM_CARDS; i++) {
deck[i].rank = (i % NUM_RANKS) + 1;
deck[i].suit = i / NUM_RANKS;
}
printf("Enter number of players: ");
scanf("%d", &num_players);
shuffle(deck); // 洗牌
// 发牌
for (i = 0; i < NUM_CARDS; i++) {
printf("Player %d: ", (i % num_players) + 1);
print_card(deck[i]);
}
return 0;
}
```
该程序使用 `card` 结构体来表示一张牌,其中 `rank` 和 `suit` 分别表示牌的点数和花色。`shuffle` 函数使用 Fisher-Yates 洗牌算法来打乱牌堆中的牌。`print_card` 函数用于打印一张牌的信息。在 `main` 函数中,程序首先初始化牌堆,然后要求用户输入参与游戏的玩家数。之后程序调用 `shuffle` 函数洗牌,并使用一个循环将每张牌发给相应的玩家。
阅读全文
相关推荐
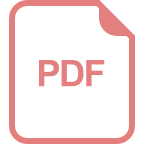
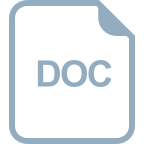
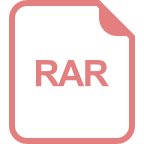















