vsc++以嵌入的资源将DLL文件打包,然后在运行时直接加载至内存,代码实现实例
时间: 2024-09-18 14:08:21 浏览: 51
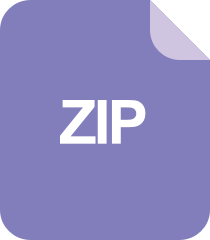
11_1__code_
在C++中,Visual Studio Code (VSC++) 不直接支持内置功能来在编译时将DLL文件打包并动态加载到内存。然而,你可以使用第三方工具如CMake、SCons或直接在代码中利用Windows API(特别是LoadLibrary函数)来实现这个过程。
以下是一个简单的例子,展示了如何在C++中通过Windows API动态加载DLL:
```cpp
#include <windows.h> // 包含Windows API头
// DLL加载函数
HMODULE LoadLibraryExample(const char* dllPath) {
HMODULE hModule = LoadLibrary(dllPath);
if (!hModule) {
std::cerr << "Failed to load library: " << GetLastError() << std::endl;
return nullptr;
}
return hModule;
}
int main() {
// 假设dllPath指向你要加载的DLL路径
const char* dllPath = "path_to_your_dll.dll";
HMODULE module = LoadLibraryExample(dllPath);
if (module) {
// 找到DLL中的某个导出函数
typedef int (*FunctionType)(int, int); // 假设你的DLL有一个名为Add的函数
FunctionType addFunc = reinterpret_cast<FunctionType>(GetProcAddress(module, "Add"));
if (addFunc) {
int result = addFunc(2, 3); // 调用函数
std::cout << "Result: " << result << std::endl;
} else {
std::cerr << "Failed to get function address" << std::endl;
}
// 释放DLL句柄
FreeLibrary(module);
} else {
std::cerr << "Failed to execute code from DLL" << std::endl;
}
return 0;
}
```
这段代码首先尝试加载DLL,然后获取其中指定函数的地址,并执行该函数。注意你需要替换`dllPath`为实际的DLL路径。
如果你希望在构建过程中进行这样的操作,可以考虑使用CMake或SCons等构建工具配合Visual Studio生成器来配置项目的链接选项,确保包含DLL,并将其静态链接或设置为运行时动态链接。
阅读全文
相关推荐
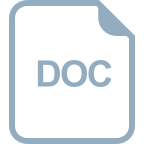
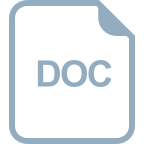
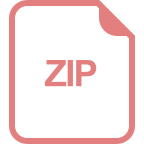
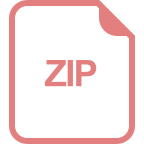
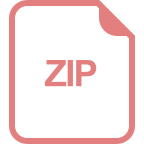












