c代码,linux系统如何为某个线程创建循环定时器
时间: 2024-10-19 10:15:40 浏览: 34
在Linux系统中,可以使用POSIX线程库(pthread)和time.h头文件来为线程创建循环定时器。以下是一个简单的示例,展示了如何在一个新线程中设置一个定期执行的任务:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <time.h>
// 定义线程函数,这里我们简单地打印一条消息并睡眠一段时间
void* timer_thread(void *arg) {
int delay = *(int*) arg; // 获取传入的延迟时间
while (1) {
printf("Timer event at %ld\n", time(NULL));
sleep(delay); // 睡眠指定秒数
}
pthread_exit(NULL);
}
// 主函数
int main() {
pthread_t thread_id;
int delay = 5; // 每5秒执行一次
if (pthread_create(&thread_id, NULL, timer_thread, &delay)) {
perror("Error creating thread");
return -1;
}
// 等待线程结束
pthread_join(thread_id, NULL);
return 0;
}
```
在这个例子中,`timer_thread`函数会被一个新的线程执行,它会无限循环地睡眠然后打印当前时间。主函数通过`pthread_create`创建线程,并将`delay`作为参数传递给线程。
注意,这只是一个基本示例,实际应用中可能需要更复杂的定时逻辑,比如处理异常、计数器等。同时,为了确保资源管理,最好在主线程中创建和销毁定时器,而不是直接在线程中操作。
阅读全文
相关推荐









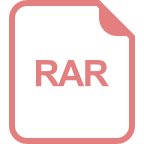
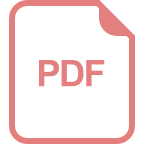

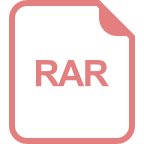





