c代码,linux系统如何基于某个线程创建循环定时器
时间: 2024-10-19 20:15:41 浏览: 34
在 Linux 系统上,我们可以使用 `librt` 库提供的 `timerfd_create()` 函数以及 `pthread` 库来基于某个线程创建循环定时器。`librt` 提供了高级的定时器 API,使得在用户空间更容易管理定时任务。
以下是一个简单的例子,展示了如何在一个新的线程中创建并启动一个循环定时器:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/time.h>
#include <unistd.h>
#include <time.h>
#include <pthread.h>
#include <sys/timerfd.h>
// 定义回调函数,这里我们简单地打印当前时间
void timer_callback(int fd, uint64_t expiring_time, int flags)
{
struct itimerspec new_value;
struct timespec now;
clock_gettime(CLOCK_MONOTONIC, &now);
printf("Timer expired at %ld.%09lu\n", now.tv_sec, now.tv_nsec);
// 如果你需要重复计时,可以重新设置定时器
if (flags == TIMER_ABSTIME) {
new_value.it_value.tv_sec = 1; // 设置新的延时秒数
new_value.it_value.tv_nsec = 0;
new_value.it_interval.tv_sec = 1;
new_value.it_interval.tv_nsec = 0;
setitimer(TIMERFD_SETTIME, &new_value, NULL); // 设置下一次定时
}
}
int main()
{
int timer_fd = timerfd_create(CLOCK_MONOTONIC, TFD_NONBLOCK | TFD_CLOEXEC);
if (timer_fd < 0) {
perror("Failed to create timer");
return -1;
}
pthread_t thread_id;
if (pthread_create(&thread_id, NULL, timer_thread, (void *)timer_fd)) {
perror("Failed to create thread");
close(timer_fd);
return -1;
}
// 等待新线程结束
pthread_join(thread_id, NULL);
close(timer_fd);
return 0;
}
// 新线程函数,用于处理定时器
void *timer_thread(void *arg)
{
int timer_fd = *(int*) arg;
struct itimerspec value;
struct timespec next_timeout;
value.it_value.tv_sec = 1; // 初始定时1秒
value.it_value.tv_nsec = 0;
value.it_interval.tv_sec = 1; // 之后每秒重复
while (true) {
if (setitimer(TIMERFD_SETTIME, &value, &next_timeout) != 0) {
perror("Error setting timer");
break;
}
// 调用回调函数
timer_callback(timer_fd, next_timeout.tv_sec + next_timeout.tv_nsec / 1e9, TIMER_ABSTIME);
// 在这里添加你的业务逻辑,比如检查条件是否满足,然后调整下次的定时值
// ...
nanosleep(&next_timeout, NULL); // 等待下一个定时
}
return NULL;
}
```
在这个示例中,`timer_thread` 线程不断地设置定时器并调用 `timer_callback`。你需要根据实际需求替换回调函数里的内容。
阅读全文
相关推荐







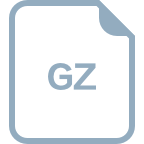

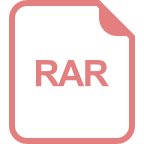


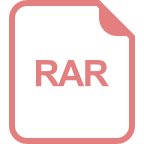





