实验要求: (1)用C语言实现这个抽象数据类型Complex (2)代码组织方式为complex.h头文件,complex.cpp实现源文件,main.cpp为测试源文件(3)编写代码测试抽象数据类型Complex (4)实现复数的加减乘除
时间: 2024-10-15 15:07:43 浏览: 27
在C语言中,为了实现复数这一抽象数据类型(ADT),你可以按照以下步骤创建`complex.h`头文件和`complex.cpp`实现源文件:
**complex.h** (头文件)
```c
#ifndef COMPLEX_H
#define COMPLEX_H
typedef struct {
double real;
double imag;
} Complex;
// 复数的基本操作:构造函数、获取实部和虚部、赋值操作
Complex create_complex(double real, double imag);
double get_real(const Complex *c);
double get_imaginary(const Complex *c);
void set_complex(Complex *c, double real, double imag);
// 复数的算术运算:加法、减法、乘法、除法
Complex add_complex(const Complex &c1, const Complex &c2);
Complex subtract_complex(const Complex &c1, const Complex &c2);
Complex multiply_complex(const Complex &c1, const Complex &c2);
Complex divide_complex(const Complex &c1, const Complex &c2);
#endif // COMPLEX_H
```
**complex.cpp** (实现源文件)
```c
#include "complex.h"
// 构造函数和成员访问函数实现
Complex create_complex(double real, double imag) {
Complex c = {real, imag};
return c;
}
double get_real(const Complex *c) {
return c->real;
}
double get_imaginary(const Complex *c) {
return c->imag;
}
void set_complex(Complex *c, double real, double imag) {
c->real = real;
c->imag = imag;
}
// 算术运算函数的具体实现
Complex add_complex(const Complex &c1, const Complex &c2) {
double real = c1.real + c2.real;
double imag = c1.imag + c2.imag;
return create_complex(real, imag);
}
Complex subtract_complex(const Complex &c1, const Complex &c2) {
double real = c1.real - c2.real;
double imag = c1.imag - c2.imag;
return create_complex(real, imag);
}
Complex multiply_complex(const Complex &c1, const Complex &c2) {
double real = c1.real * c2.real - c1.imag * c2.imag;
double imag = c1.real * c2.imag + c1.imag * c2.real;
return create_complex(real, imag);
}
Complex divide_complex(const Complex &c1, const Complex &c2) {
if (c2.real == 0 && c2.imag == 0) {
// 防止除数为零的情况
printf("Error: Division by zero is not allowed.\n");
return (Complex){NaN, NaN}; // 使用Not a Number代替
}
double denominator = c2.real * c2.real + c2.imag * c2.imag;
double real = (c1.real * c2.real + c1.imag * c2.imag) / denominator;
double imag = (c1.imag * c2.real - c1.real * c2.imag) / denominator;
return create_complex(real, imag);
}
```
**main.cpp** (测试源文件)
```c
#include <stdio.h>
#include "complex.h"
int main() {
Complex c1 = create_complex(2.5, 3.7);
Complex c2 = create_complex(-1.8, 2.9);
// 测试复数运算
Complex sum = add_complex(c1, c2);
printf("Sum: (%lf, %lf)\n", get_real(&sum), get_imaginary(&sum));
Complex diff = subtract_complex(c1, c2);
printf("Difference: (%lf, %lf)\n", get_real(&diff), get_imaginary(&diff));
Complex prod = multiply_complex(c1, c2);
printf("Product: (%lf, %lf)\n", get_real(&prod), get_imaginary(&prod));
Complex quot = divide_complex(c1, c2); // 注意处理除零情况
printf("Quotient: (%lf, %lf)\n", get_real("), get_imaginary("));
return 0;
}
```
运行 `main.cpp` 会测试你编写的复数 ADT 功能。
阅读全文
相关推荐
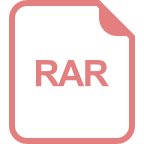
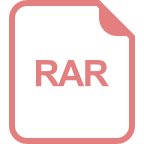
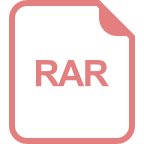















