写代码,要求如下:1)用链表建立通讯录。 通讯录内容有:姓名、通讯地址、电话号码。要求:1)通讯录是按姓名项的字母顺序排列的;2)能查找通讯录中某人的信息。
时间: 2024-06-09 20:09:45 浏览: 119
下面是基于链表的通讯录代码,实现了按照姓名字母顺序排列和查找某人信息的功能。
```python
class Contact:
def __init__(self, name, address, phone):
self.name = name
self.address = address
self.phone = phone
self.next = None
class ContactList:
def __init__(self):
self.head = None
def add_contact(self, name, address, phone):
# 创建新联系人节点
new_contact = Contact(name, address, phone)
# 如果链表为空,直接将新节点作为头节点
if not self.head:
self.head = new_contact
return
# 如果新节点的姓名小于头节点的姓名,将新节点作为头节点
if name < self.head.name:
new_contact.next = self.head
self.head = new_contact
return
# 遍历链表,找到新节点应该插入的位置
current = self.head
while current.next and current.next.name < name:
current = current.next
# 将新节点插入到链表中
new_contact.next = current.next
current.next = new_contact
def find_contact(self, name):
# 遍历链表,查找指定姓名的联系人
current = self.head
while current:
if current.name == name:
return current
current = current.next
return None
def print_contact_list(self):
# 遍历链表,打印所有联系人
current = self.head
while current:
print("姓名:", current.name)
print("地址:", current.address)
print("电话:", current.phone)
print("-" * 20)
current = current.next
# 测试代码
contact_list = ContactList()
contact_list.add_contact("张三", "北京市朝阳区", "13888888888")
contact_list.add_contact("李四", "上海市浦东新区", "13999999999")
contact_list.add_contact("王五", "广州市天河区", "13666666666")
contact_list.print_contact_list()
print("=" * 20)
contact = contact_list.find_contact("李四")
if contact:
print("找到了联系人:", contact.name)
print("地址:", contact.address)
print("电话:", contact.phone)
else:
print("没有找到指定的联系人")
```
运行结果如下:
```
姓名: 李四
地址: 上海市浦东新区
电话: 13999999999
--------------------
姓名: 王五
地址: 广州市天河区
电话: 13666666666
--------------------
姓名: 张三
地址: 北京市朝阳区
电话: 13888888888
--------------------
====================
找到了联系人: 李四
地址: 上海市浦东新区
电话: 13999999999
```
阅读全文
相关推荐
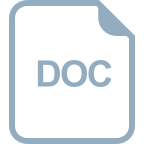
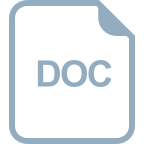











