五子棋python悔棋
时间: 2024-12-30 18:25:31 浏览: 6
### Python 实现五子棋悔棋功能
为了实现五子棋游戏中的悔棋功能,可以通过记录每一步的操作历史来完成。每当玩家希望撤销上一次移动时,程序会从操作栈中弹出最近的一次落子位置,并更新棋盘状态。
下面是一个简单的Python代码示例,展示了如何使用列表作为堆栈结构存储每次下棋的位置以及对应的玩家编号:
```python
class GomokuGame:
def __init__(self):
self.board = [[0 for _ in range(15)] for _ in range(15)]
self.history = [] # 记录走法的历史
self.current_player = 1 # 默认黑方先行
def place_chess(self, x, y):
"""放置棋子"""
if not (0 <= x < 15 and 0 <= y < 15) or self.board[x][y] != 0:
raise ValueError('Invalid move')
self.board[x][y] = self.current_player
self.history.append((x, y))
self._switch_player()
def undo_move(self):
"""撤回最后一步"""
if len(self.history) == 0:
print("No moves to undo.")
return False
last_x, last_y = self.history.pop()
self.board[last_x][last_y] = 0
self._switch_player() # 切换回之前的玩家
return True
def _switch_player(self):
"""切换当前玩家"""
self.current_player = 3 - self.current_player # 如果是1则变为2;如果是2则变为1
def display_board(self):
"""打印棋盘"""
for row in self.board:
print(row)
game = GomokuGame()
game.place_chess(7, 7)
game.display_board()
print("\nAfter Undo:")
game.undo_move()
game.display_board()
```
这段代码定义了一个`GomokuGame`类,其中包含了放置棋子(`place_chess`)、撤回上一着(`undo_move`)的方法[^4]。通过这种方式,不仅可以轻松地管理用户的输入,还能有效地处理复杂的局面变化。
阅读全文
相关推荐










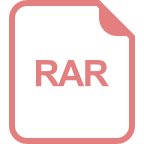







