用Python的opcv绘制一个像素大小为 100x240 的圆角矩形,圆角半径像素为 20
时间: 2024-10-13 15:05:29 浏览: 45
在Python中,我们可以使用OpenCV (Open Source Computer Vision Library) 的 `cv2` 模块来绘制带圆角的矩形。首先,需要安装OpenCV库,如果没有安装可以使用pip进行安装:
```bash
pip install opencv-python
```
下面是一个示例代码片段,它会创建一个100x240像素的圆角矩形,圆角半径为20像素:
```python
import cv2
import numpy as np
# 创建一个黑色背景的图像
img = np.zeros((240, 100, 3), dtype=np.uint8)
# 设置圆角矩形的尺寸和圆角半径
width = 100
height = 240
radius = 20
# 计算实际的矩形宽度和高度,考虑到圆角部分会被占用
rect_width = width + 2 * radius
rect_height = height + 2 * radius
# 定义顶点坐标,用于计算轮廓和填充区域
vertices = np.array([[(0, rect_height - radius),
(radius, rect_height),
(width - radius, rect_height),
(width, rect_height - radius)],
[(0, radius),
(radius, 0),
(width - radius, 0),
(width, radius)]], np.int32)
# 使用fillPoly函数填充形状,然后应用mask
mask = np.zeros(img.shape[:2], np.uint8)
cv2.fillConvexPoly(mask, vertices, (255, 255, 255)) # 填充白色
img[mask == 255] = [0, 0, 0] # 将mask区域设置回黑色
# 显示结果
cv2.imshow('Rounded Rectangle', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
当你运行这个程序时,它会在窗口中显示一个100x240像素的带圆角的矩形。
阅读全文
相关推荐
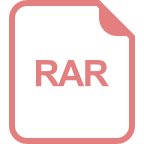
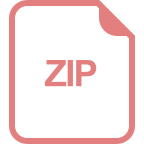
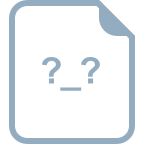



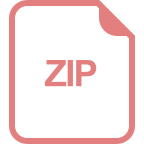
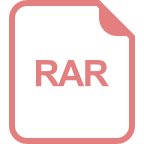
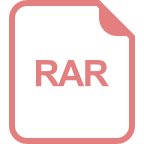







