jupyter+notebook中str.split
时间: 2023-12-25 22:29:14 浏览: 74
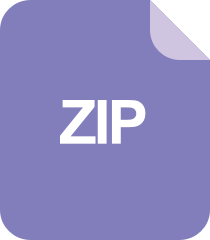
jupyter_in_streamlit
在Jupyter Notebook中,可以使用str.split()方法将字符串拆分为多个子字符串,并返回一个列表。该方法可以接受一个可选的参数,用于指定分隔符,默认情况下使用空格作为分隔符。
以下是一个示例演示如何在Jupyter Notebook中使用str.split()方法:
```python
# 定义一个字符串
string = "Hello World"
# 使用split()方法拆分字符串
result = string.split()
# 打印拆分后的结果
print(result) # 输出:['Hello', 'World']
```
在上述示例中,我们定义了一个字符串"Hello World",然后使用split()方法将其拆分为两个子字符串,并将结果存储在一个列表中。最后,我们打印出拆分后的结果。
阅读全文
相关推荐
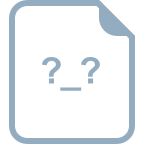
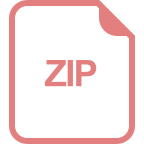
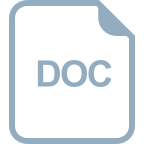
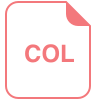
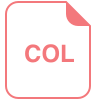
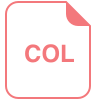
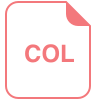
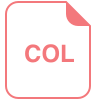









