数据结构的形式c语言一元多项式求和
时间: 2024-10-11 19:02:39 浏览: 7
在 C 语言中,我们可以使用数组和指针来表示一元多项式的各个系数。一元多项式通常表示为形如 `a_n * x^n + a_{n-1} * x^{n-1} + ... + a_1 * x^1 + a_0` 的形式,其中 `n` 是最高次幂,`a_i` 是对应的系数。
下面是一个简单的例子,我们创建一个数据结构来存储一元多项式的系数及其指数:
```c
typedef struct {
int exponent; // 系数对应的指数
double coefficient; // 系数值
} PolynomialTerm;
typedef struct {
int degree; // 最高次幂
PolynomialTerm terms[1]; // 动态数组,用于存储多项式系数
} Polynomial;
```
然后,我们可以编写函数来初始化多项式、添加新项以及求和两个多项式:
```c
// 初始化多项式
void initPolynomial(Polynomial* poly, int n) {
poly->degree = n;
}
// 添加新项
void addTerm(Polynomial* poly, int exp, double coef) {
if (poly->degree < exp) { // 如果新项的指数大于现有最大指数,则扩大数组容量
poly->degree = exp + 1;
poly->terms = realloc(poly->terms, sizeof(PolynomialTerm) * (poly->degree + 1));
}
poly->terms[poly->degree].exponent = exp;
poly->terms[poly->degree].coefficient = coef;
}
// 求两个多项式的和
Polynomial sumPolynomials(const Polynomial* poly1, const Polynomial* poly2) {
Polynomial result;
initPolynomial(&result, MAX(poly1->degree, poly2->degree)); // 取较大度数作为新多项式度
for (int i = 0; i <= result.degree; ++i) {
result.terms[i].coefficient += poly1->terms[i].coefficient + poly2->terms[i].coefficient;
}
return result;
}
```
相关推荐
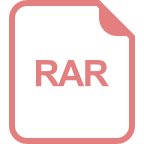
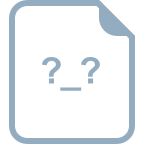
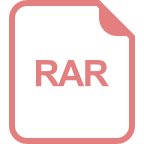
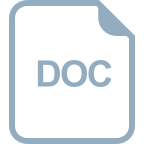
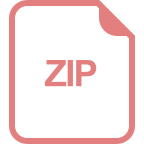
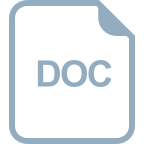
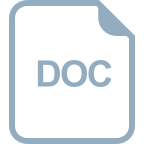
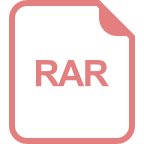
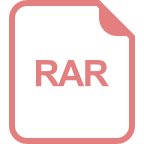
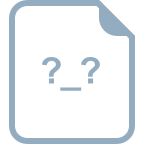
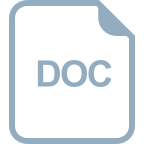
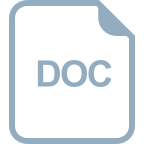
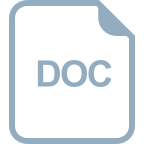
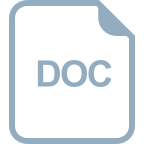

